用C++写一个程序可以从键盘输入任意多个英语单词,中间用空格分开,输入0表示输入结束。程序会统计同一个英语单词被输入的次数,最后对英文单词按字典顺序输出,后面跟上该单词被输入的次数。程序使用结构体来组织数据,把单词和该单词出现的次数用一个结构体来描述。
时间: 2023-05-20 17:03:37 浏览: 112
好的,这是一个非常基础的单词计数程序,以下是代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_WORD_LEN 50
#define MAX_WORDS 1000
struct word_count {
char word[MAX_WORD_LEN];
int count;
};
int main() {
struct word_count words[MAX_WORDS];
int num_words = 0;
// 读入单词
char word[MAX_WORD_LEN];
while (scanf("%s", word) == 1 && strcmp(word, "0") != 0) {
// 检查单词是否已经存在
int i;
for (i = 0; i < num_words; i++) {
if (strcmp(words[i].word, word) == 0) {
words[i].count++;
break;
}
}
// 如果单词不存在,则添加到数组中
if (i == num_words) {
strcpy(words[num_words].word, word);
words[num_words].count = 1;
num_words++;
}
}
// 按字典顺序排序
int i, j;
for (i = 0; i < num_words - 1; i++) {
for (j = i + 1; j < num_words; j++) {
if (strcmp(words[i].word, words[j].word) > 0) {
struct word_count temp = words[i];
words[i] = words[j];
words[j] = temp;
}
}
}
// 输出结果
for (i = 0; i < num_words; i++) {
printf("%s %d\n", words[i].word, words[i].count);
}
return 0;
}
```
这个程序使用了一个结构体 `word_count` 来存储单词和它出现的次数,同时使用一个数组 `words` 来存储所有的单词。程序首先读入单词,然后检查单词是否已经存在于数组中,如果存在,则增加它的计数器,否则将它添加到数组中。最后,程序按字典顺序对数组进行排序,并输出结果。
阅读全文
相关推荐
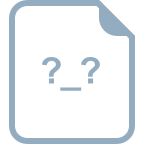
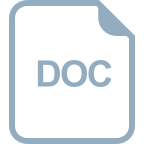
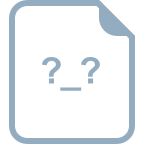
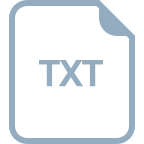
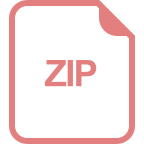
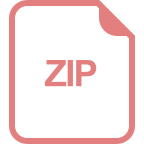
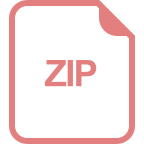
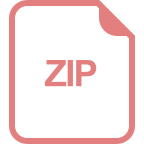
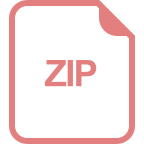
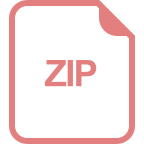