用java定义员工类Teacher类,包括成员变量name,sno,education,构造方法Teacher(String name,String sno,String education),并为三个成员变量设置对应的三对get、set方法,重写toString方法用于获取教师信息;2、让用户在控制台输入教师的数量,比方说输入5,则需要输入个教师的姓名、工号、学历,并构造对应的教师对象,把教师对象存入HashMap集合完毕中,并同时存入一个文件中,教师信息录入完毕后,从文件中读出所有的教师信息
时间: 2024-01-07 11:05:33 浏览: 139
好的,这是您的问题:
1. 用java定义员工类Teacher类,包括成员变量name,sno,education,构造方法Teacher(String name,String sno,String education),并为三个成员变量设置对应的三对get、set方法,重写toString方法用于获取教师信息;
2. 让用户在控制台输入教师的数量,比方说输入5,则需要输入个教师的姓名、工号、学历,并构造对应的教师对象,把教师对象存入HashMap集合完毕中,并同时存入一个文件中,教师信息录入完毕后,从文件中读出所有的教师信息。
下面是Java的实现代码:
```
import java.io.*;
import java.util.*;
public class Teacher {
private String name;
private String sno;
private String education;
public Teacher(String name, String sno, String education) {
this.name = name;
this.sno = sno;
this.education = education;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSno() {
return sno;
}
public void setSno(String sno) {
this.sno = sno;
}
public String getEducation() {
return education;
}
public void setEducation(String education) {
this.education = education;
}
@Override
public String toString() {
return "姓名:" + this.name + ",工号:" + this.sno + ",学历:" + this.education;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入教师数量:");
int n = scanner.nextInt();
scanner.nextLine(); // 消费掉输入的回车
// 存储教师信息的HashMap
HashMap<String, Teacher> teachers = new HashMap<>();
// 创建文件
File file = new File("teachers.txt");
try {
file.createNewFile();
FileWriter writer = new FileWriter(file);
for (int i = 0; i < n; i++) {
System.out.println("请输入教师姓名:");
String name = scanner.nextLine();
System.out.println("请输入教师工号:");
String sno = scanner.nextLine();
System.out.println("请输入教师学历:");
String education = scanner.nextLine();
Teacher teacher = new Teacher(name, sno, education);
teachers.put(sno, teacher);
writer.write(teacher.toString() + "\n");
}
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
// 读取文件中的教师信息
try {
FileReader reader = new FileReader(file);
BufferedReader bufferedReader = new BufferedReader(reader);
String line;
while ((line = bufferedReader.readLine()) != null) {
System.out.println(line);
}
bufferedReader.close();
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这段代码定义了一个`Teacher`类,包含三个成员变量`name`、`sno`和`education`,以及对应的`get`、`set`方法和`toString`方法。在`main`函数中,先让用户输入教师数量,然后循环读取每个教师的姓名、工号和学历,并构造对应的`Teacher`对象,在`HashMap`中存储教师信息,并将教师信息写入到文件`teachers.txt`中。最后再从文件中读取所有的教师信息,输出到控制台上。
阅读全文
相关推荐
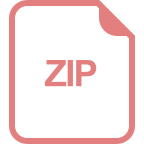
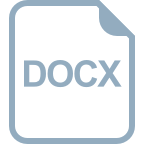
















