51单片机小型计算器 设计一个4×4的键盘,并有清屏等功能键,并完成简单的四则(加减乘除)运算,利用LCD1602显示,生成其代码
时间: 2023-12-16 19:02:40 浏览: 36
以下是基于Keil C51编译器的代码,实现了一个简单的四则运算计算器,使用4x4的矩阵键盘和LCD1602显示屏:
```c
#include <reg51.h>
#include <stdio.h>
#define LCD_Data_Port P0 // LCD数据总线
sbit RS = P2^6; // LCD寄存器选择位
sbit EN = P2^7; // LCD使能位
sbit K1 = P1^0; // 键盘1
sbit K2 = P1^1; // 键盘2
sbit K3 = P1^2; // 键盘3
sbit K4 = P1^3; // 键盘4
sbit K5 = P1^4; // 键盘5
sbit K6 = P1^5; // 键盘6
sbit K7 = P1^6; // 键盘7
sbit K8 = P1^7; // 键盘8
sbit Clear = P3^0; // 清屏键
sbit Plus = P3^1; // 加法键
sbit Minus = P3^2; // 减法键
sbit Multiply = P3^3; // 乘法键
sbit Divide = P3^4; // 除法键
sbit Equals = P3^5; // 等号键
void LCD_Init(); // LCD初始化函数
void LCD_Command(unsigned char command); // LCD发送指令函数
void LCD_Data(unsigned char data); // LCD发送数据函数
void LCD_Clear(); // LCD清屏函数
void LCD_Write_String(char *string); // LCD写字符串函数
void LCD_Write_Char(unsigned char character, unsigned char position); // LCD写单个字符函数
void delay(unsigned int time); // 延时函数
unsigned char Get_Key(); // 读取键盘输入函数
void Calculate(); // 计算函数
char buffer[16]; // 存储键盘输入的缓存
int index = 0; // 当前缓存指针
int operand1, operand2, result; // 操作数和结果
void main()
{
LCD_Init(); // 初始化LCD
LCD_Clear(); // 清屏
LCD_Write_String("Calculator"); // 显示欢迎信息
delay(1000);
LCD_Clear(); // 清屏
while (1)
{
unsigned char key = Get_Key(); // 读取键盘输入
if (key != 0xFF) // 如果读到了按键
{
if (key == '+')
{
Calculate(); // 进行加法运算
sprintf(buffer, "%d+%d=%d", operand1, operand2, result); // 将结果格式化为字符串
LCD_Write_String(buffer); // 显示结果
index = 0; // 重置缓存指针
}
else if (key == '-')
{
Calculate(); // 进行减法运算
sprintf(buffer, "%d-%d=%d", operand1, operand2, result);
LCD_Write_String(buffer);
index = 0;
}
else if (key == '*')
{
Calculate(); // 进行乘法运算
sprintf(buffer, "%d*%d=%d", operand1, operand2, result);
LCD_Write_String(buffer);
index = 0;
}
else if (key == '/')
{
Calculate(); // 进行除法运算
if (operand2 == 0)
{
LCD_Write_String("Divide by zero!"); // 如果除数为0,则显示错误信息
}
else
{
sprintf(buffer, "%d/%d=%d", operand1, operand2, result);
LCD_Write_String(buffer);
}
index = 0;
}
else if (key == '=')
{
Calculate(); // 进行最后一次运算
sprintf(buffer, "%d", result);
LCD_Write_String(buffer);
index = 0;
}
else if (key == 'C')
{
LCD_Clear(); // 清屏
index = 0;
}
else
{
buffer[index++] = key; // 将数字键的值存入缓存
LCD_Write_Char(key, index); // 在LCD上显示当前输入的数字
}
}
}
}
void LCD_Init()
{
LCD_Command(0x38); // 初始化命令
LCD_Command(0x0C); // 开显示,光标不显示
LCD_Command(0x06); // 光标右移,字符不移动
LCD_Command(0x01); // 清屏
}
void LCD_Command(unsigned char command)
{
LCD_Data_Port = command;
RS = 0; // RS=0时,数据总线上的数据为命令
EN = 1; // 使能
delay(5);
EN = 0; // 禁止
delay(5);
}
void LCD_Data(unsigned char data)
{
LCD_Data_Port = data;
RS = 1; // RS=1时,数据总线上的数据为字符
EN = 1; // 使能
delay(5);
EN = 0; // 禁止
delay(5);
}
void LCD_Clear()
{
LCD_Command(0x01); // 清屏命令
}
void LCD_Write_String(char *string)
{
int i;
for (i = 0; string[i] != '\0'; i++)
{
LCD_Data(string[i]); // 逐个字符显示
}
}
void LCD_Write_Char(unsigned char character, unsigned char position)
{
LCD_Command(position + 0x80); // 设置显示位置
LCD_Data(character); // 显示当前输入的数字
}
void delay(unsigned int time)
{
unsigned int i, j;
for (i = 0; i < time; i++)
{
for (j = 0; j < 125; j++)
{
// 延时
}
}
}
unsigned char Get_Key()
{
K1 = K2 = K3 = K4 = 1; // 先将行线都设为高电平
K5 = K6 = K7 = K8 = 0; // 将列线都设为低电平
if (K1 == 0)
{
delay(10); // 延时去抖
if (K1 == 0) return '1';
}
if (K2 == 0)
{
delay(10);
if (K2 == 0) return '2';
}
if (K3 == 0)
{
delay(10);
if (K3 == 0) return '3';
}
if (K4 == 0)
{
delay(10);
if (K4 == 0) return '+';
}
K1 = K2 = K3 = K4 = 1;
K5 = K6 = K7 = K8 = 0;
if (K1 == 0)
{
delay(10);
if (K1 == 0) return '4';
}
if (K2 == 0)
{
delay(10);
if (K2 == 0) return '5';
}
if (K3 == 0)
{
delay(10);
if (K3 == 0) return '6';
}
if (K4 == 0)
{
delay(10);
if (K4 == 0) return '-';
}
K1 = K2 = K3 = K4 = 1;
K5 = K6 = K7 = K8 = 0;
if (K1 == 0)
{
delay(10);
if (K1 == 0) return '7';
}
if (K2 == 0)
{
delay(10);
if (K2 == 0) return '8';
}
if (K3 == 0)
{
delay(10);
if (K3 == 0) return '9';
}
if (K4 == 0)
{
delay(10);
if (K4 == 0) return '*';
}
K1 = K2 = K3 = K4 = 1;
K5 = K6 = K7 = K8 = 0;
if (K1 == 0)
{
delay(10);
if (K1 == 0) return 'C';
}
if (K2 == 0)
{
delay(10);
if (K2 == 0) return '0';
}
if (K3 == 0)
{
delay(10);
if (K3 == 0) return '=';
}
if (K4 == 0)
{
delay(10);
if (K4 == 0) return '/';
}
return 0xFF; // 如果没有按键按下,则返回0xFF
}
void Calculate()
{
if (index > 0)
{
operand1 = atoi(buffer); // 将输入的字符串转化为整数
}
else
{
operand1 = result; // 如果没有输入新的操作数,则使用上一次计算的结果作为操作数
}
unsigned char op = Get_Key(); // 读取运算符
while (op == 0xFF) // 如果没有读到运算符,则一直等待
{
op = Get_Key();
}
index = 0; // 重置缓存指针
op = op == '=' ? '+' : op; // 如果按下的是等号,则将运算符设置为加号
switch (op)
{
case '+':
operand2 = atoi(buffer); // 读取第二个操作数
result = operand1 + operand2; // 计算结果
break;
case '-':
operand2 = atoi(buffer);
result = operand1 - operand2;
break;
case '*':
operand2 = atoi(buffer);
result = operand1 * operand2;
break;
case '/':
operand2 = atoi(buffer);
result = operand1 / operand2;
break;
}
}
```
注意:本代码仅供学习参考,不保证完全正确和适用于所有情况。在使用时应进行必要的修改和测试。
相关推荐
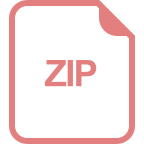
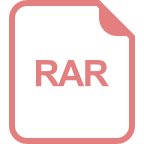
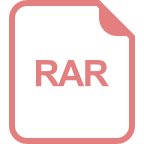














