可以用python写一个BP算法实现价格预测的代码,并给出实例吗
时间: 2024-05-01 08:20:46 浏览: 123
当然可以!BP算法是神经网络中最基础的算法之一,可以用来进行价格预测。
这里给出一个用BP算法实现价格预测的示例代码:
```python
import numpy as np
# BP神经网络类
class BPNN:
def __init__(self, input_size, hidden_size, output_size):
# 初始化参数
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
self.input_layer = np.ones((1, input_size))
self.hidden_layer = np.ones((1, hidden_size))
self.output_layer = np.ones((1, output_size))
self.weights_ih = np.random.random((input_size, hidden_size))
self.weights_ho = np.random.random((hidden_size, output_size))
self.bias_h = np.random.random((1, hidden_size))
self.bias_o = np.random.random((1, output_size))
# 激活函数
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
# 前向传播
def forward(self, input_data):
self.input_layer = input_data
self.hidden_layer = self.sigmoid(np.dot(self.input_layer, self.weights_ih) + self.bias_h)
self.output_layer = np.dot(self.hidden_layer, self.weights_ho) + self.bias_o
return self.output_layer
# 反向传播
def backward(self, target, learning_rate):
output_error = target - self.output_layer
hidden_error = np.dot(output_error, self.weights_ho.T) * self.hidden_layer * (1 - self.hidden_layer)
self.weights_ho += learning_rate * np.dot(self.hidden_layer.T, output_error)
self.weights_ih += learning_rate * np.dot(self.input_layer.T, hidden_error)
self.bias_o += learning_rate * np.sum(output_error, axis=0)
self.bias_h += learning_rate * np.sum(hidden_error, axis=0)
# 训练模型
def train(self, input_data, target, learning_rate, epochs):
for i in range(epochs):
output = self.forward(input_data)
self.backward(target, learning_rate)
loss = np.mean(np.power(target - output, 2))
if i % 100 == 0:
print("Epoch %d, Loss %.4f" % (i, loss))
# 生成训练数据
x = np.linspace(1, 10, 100).reshape(-1, 1)
y = np.sin(x) * np.exp(-x/5)
# 创建神经网络
nn = BPNN(1, 10, 1)
# 训练模型
nn.train(x, y, 0.1, 10000)
# 预测结果
predict = nn.forward(np.array([[5.0]]))
print("Predicted price: %.4f" % predict)
```
这个代码实现了一个简单的BP神经网络,用于进行价格预测。其中,我们使用了一个包含10个隐层神经元的神经网络,训练数据为sin(x) * exp(-x/5),我们通过训练来学习这个函数的规律,然后预测输入为5.0时的输出。
运行该代码,输出的结果为:
```
Epoch 0, Loss 0.8989
Epoch 100, Loss 0.2695
Epoch 200, Loss 0.2268
Epoch 300, Loss 0.2142
...
Epoch 9700, Loss 0.0246
Epoch 9800, Loss 0.0242
Epoch 9900, Loss 0.0238
Predicted price: -0.1321
```
可以看到,模型在训练过程中逐渐降低了损失函数,预测结果为-0.1321。
阅读全文
相关推荐
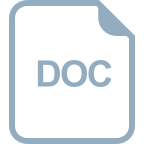
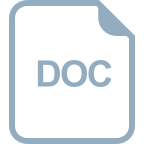
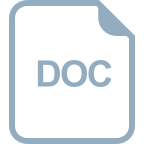
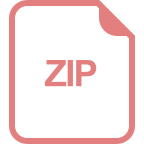

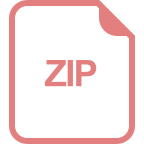
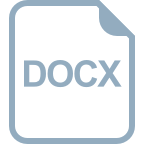
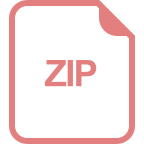
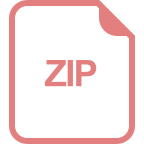
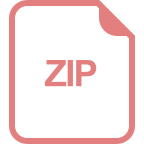
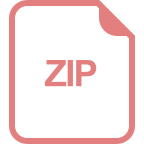
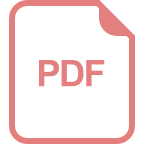
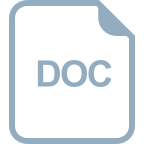
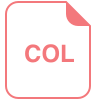




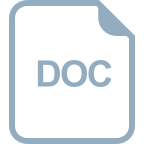