给我一段K-shell算法的代码
时间: 2024-06-11 16:04:51 浏览: 86
由于您没有具体说明K-shell算法的具体实现方式,以下是一种常见的K-shell算法实现方式的代码示例:
```
// K-shell algorithm implementation in C++
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
vector<int> k_shell_algorithm(vector<vector<int>> graph, int k) {
int n = graph.size();
vector<int> degrees(n, 0);
vector<vector<int>> adj_list(n, vector<int>());
// Calculate degrees of all vertices and create adjacency list
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
if (graph[i][j] == 1) {
degrees[i]++;
adj_list[i].push_back(j);
}
}
}
// Create queue and initialize with vertices having degree less than k
queue<int> q;
for (int i = 0; i < n; i++) {
if (degrees[i] < k) {
q.push(i);
degrees[i] = -1; // Mark vertex as visited
}
}
// Remove vertices with degree less than k and update degrees of their neighbors
while (!q.empty()) {
int u = q.front();
q.pop();
for (int v : adj_list[u]) {
if (degrees[v] > 0) {
degrees[v]--;
if (degrees[v] < k) {
q.push(v);
degrees[v] = -1; // Mark vertex as visited
}
}
}
}
// Collect vertices with degree greater than or equal to k
vector<int> k_shell_vertices;
for (int i = 0; i < n; i++) {
if (degrees[i] >= k) {
k_shell_vertices.push_back(i);
}
}
return k_shell_vertices;
}
int main() {
// Example usage
vector<vector<int>> graph = {{0, 1, 1, 0, 0},
{1, 0, 1, 1, 0},
{1, 1, 0, 0, 1},
{0, 1, 0, 0, 1},
{0, 0, 1, 1, 0}};
int k = 3;
vector<int> k_shell_vertices = k_shell_algorithm(graph, k);
cout << "Vertices in " << k << "-shell: ";
for (int v : k_shell_vertices) {
cout << v << " ";
}
cout << endl;
return 0;
}
```
该代码实现了一个基于邻接矩阵的K-shell算法,其中k_shell_algorithm函数接受一个邻接矩阵和一个整数k作为输入,返回一个包含所有K-shell上的顶点的向量。该算法的核心是通过迭代删除度数小于k的顶点及其相邻的边来计算K-shell。在代码中,我们使用了一个队列来存储需要删除的节点,同时使用了一个度数向量来记录每个节点的度数以及标记已经访问过的节点。
阅读全文
相关推荐

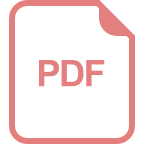
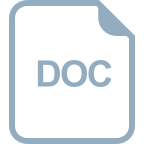
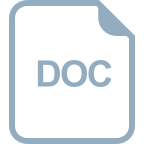
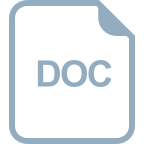
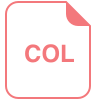
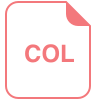
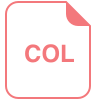






