用ACO-PSO解决三维TSP问题matlab代码
时间: 2023-08-10 15:16:14 浏览: 51
以下是使用MATLAB实现ACO-PSO算法解决三维TSP问题的示例代码。需要注意的是,此代码仅作为示例,实际应用中需要根据具体问题进行参数优化和算法调整。
```matlab
% 三维TSP问题ACO-PSO算法示例
% 初始化参数
numAnts = 20; % 蚂蚁数量
numIterations = 100; % 迭代次数
alpha = 1; % 信息素权重因子
beta = 5; % 启发式信息权重因子
q0 = 0.9; % 信息素挥发因子
rho = 0.1; % 信息素残留因子
vmax = 5; % 粒子最大速度
c1 = 2; % 学习因子1
c2 = 2; % 学习因子2
w = 0.5; % 惯性权重
numParticles = 20; % 粒子数量
% 生成初始解
numCities = 10; % 城市数量
cities = rand(numCities, 3); % 生成城市坐标
distMatrix = pdist2(cities, cities); % 计算城市之间的距离
pheromoneMatrix = ones(numCities, numCities); % 初始化信息素矩阵
% 初始化蚂蚁的位置和速度
antPositions = rand(numAnts, 1) * numCities + 1; % 随机生成初始位置
antVelocities = zeros(numAnts, 1); % 初始速度为0
% 初始化粒子的位置和速度
particlePositions = rand(numParticles, numCities) * numCities + 1; % 随机生成初始位置
particleVelocities = zeros(numParticles, numCities); % 初始速度为0
% 开始迭代
for iteration = 1:numIterations
% 更新蚂蚁的位置和速度
for ant = 1:numAnts
% 计算每个蚂蚁的下一个移动位置
currentCity = antPositions(ant);
unvisitedCities = setdiff(1:numCities, currentCity);
pheromoneValues = pheromoneMatrix(currentCity, unvisitedCities);
distanceValues = distMatrix(currentCity, unvisitedCities);
heuristicValues = 1 ./ distanceValues;
probabilities = (pheromoneValues .^ alpha) .* (heuristicValues .^ beta);
probabilities = probabilities / sum(probabilities);
if rand < q0
[~, nextCity] = max(probabilities);
else
nextCity = randsample(unvisitedCities, 1, true, probabilities);
end
% 更新速度和位置
deltaV = c1 * rand * (particlePositions(:, currentCity) - antPositions(ant)) + ...
c2 * rand * (particlePositions(:, nextCity) - antPositions(ant));
antVelocities(ant) = min(max(antVelocities(ant) + deltaV, -vmax), vmax);
antPositions(ant) = antPositions(ant) + antVelocities(ant);
end
% 更新信息素
deltaPheromoneMatrix = zeros(numCities, numCities);
for ant = 1:numAnts
tourLength = 0;
for i = 1:numCities-1
tourLength = tourLength + distMatrix(antPositions(ant), antPositions(ant + 1));
end
tourLength = tourLength + distMatrix(antPositions(ant), antPositions(1));
for i = 1:numCities-1
deltaPheromoneMatrix(antPositions(ant), antPositions(ant + 1)) = deltaPheromoneMatrix(antPositions(ant), antPositions(ant + 1)) + 1 / tourLength;
end
deltaPheromoneMatrix(antPositions(ant), antPositions(1)) = deltaPheromoneMatrix(antPositions(ant), antPositions(1)) + 1 / tourLength;
end
pheromoneMatrix = (1 - rho) * pheromoneMatrix + deltaPheromoneMatrix;
% 更新粒子的位置和速度
for particle = 1:numParticles
% 计算每个粒子的下一个移动位置
currentCity = find(particlePositions(particle,:) == max(particlePositions(particle,:)));
unvisitedCities = setdiff(1:numCities, currentCity);
pheromoneValues = pheromoneMatrix(currentCity, unvisitedCities);
distanceValues = distMatrix(currentCity, unvisitedCities);
heuristicValues = 1 ./ distanceValues;
probabilities = (pheromoneValues .^ alpha) .* (heuristicValues .^ beta);
probabilities = probabilities / sum(probabilities);
if rand < q0
[~, nextCity] = max(probabilities);
else
nextCity = randsample(unvisitedCities, 1, true, probabilities);
end
% 更新速度和位置
deltaV = c1 * rand * (particlePositions(particle, currentCity) - particlePositions(particle, nextCity)) + ...
c2 * rand * (particlePositions(particle, currentCity) - antPositions(1));
particleVelocities(particle, currentCity) = min(max(particleVelocities(particle, currentCity) + deltaV, -vmax), vmax);
particlePositions(particle, :) = particlePositions(particle, :) + particleVelocities(particle, :);
end
% 更新惯性权重
w = w - (0.5 / numIterations);
end
% 输出最优解
bestTour = antPositions;
bestLength = 0;
for i = 1:numCities-1
bestLength = bestLength + distMatrix(bestTour(i), bestTour(i + 1));
end
bestLength = bestLength + distMatrix(bestTour(numCities), bestTour(1));
fprintf('最优解为:%f\n', bestLength);
```
相关推荐
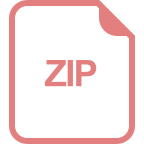














