用python的scikit_learn写一个OPTICS聚类算法
时间: 2024-04-29 09:26:37 浏览: 10
可以的,请看下面的代码:
```python
from sklearn.cluster import OPTICS
import numpy as np
# 准备数据
X = np.array([[1, 2], [1, 4], [1, 0], [4, 2], [4, 4], [4, 0]])
# 调用OPTICS算法
clustering = OPTICS(min_samples=2).fit(X)
# 打印聚类结果
print(clustering.labels_)
```
这里的 `X` 表示原始数据,`min_samples` 表示一个聚类中最少有多少个样本点。最后 `clustering.labels_` 打印的是每个样本点所属的簇的标签。
相关问题
optics聚类算法 python
Optics 聚类算法是一种基于密度的聚类算法,它可以处理任意形状的簇,并且不需要预设簇的数量。在 Python 中,可以使用 Scikit-learn 库来实现 Optics 聚类算法。具体实现步骤包括数据预处理、构建 Optics 对象、拟合数据和可视化聚类结果等。以下是一个简单的示例代码:
```python
from sklearn.cluster import OPTICS
# 准备数据
X = [[2, 4], [2, 5], [4, 6], [4, 7], [4, 8], [6, 9], [6, 10], [7, 8]]
# 构建 Optics 对象
optics_model = OPTICS(min_samples=2, xi=0.05)
# 拟合数据
optics_model.fit(X)
# 可视化聚类结果
import matplotlib.pyplot as plt
labels = optics_model.labels_
reachability = optics_model.reachability_
plt.figure(figsize=(10, 7))
plt.title('Optics Clustering')
plt.scatter(X[:,0], X[:,1], c=labels, cmap='viridis')
plt.show()
```
该示例代码使用了一个简单的二维数据集,构建 Optics 对象时设置了最小样本数为 2,$\xi$ 参数为 0.05。最后,可视化聚类结果并展示给用户。
optics聚类算法
OPTICS聚类算法是一种基于密度的聚类算法,它是DBSCAN算法的扩展。OPTICS算法通过计算每个数据点的可达距离和核心距离来确定数据点的聚类关系。可达距离表示一个数据点到其他数据点的最小距离,核心距离表示一个数据点的邻域内的最小距离。
OPTICS算法的主要思想是根据可达距离和核心距离构建一个可达距离图,然后通过遍历图的节点来确定聚类结果。算法首先将数据点按照可达距离进行排序,然后从第一个数据点开始,依次计算每个数据点的核心距离和可达距离。根据核心距离和可达距离的关系,可以确定数据点的聚类关系,包括核心点、边界点和噪声点。
在scikit-learn中,可以使用OPTICS聚类算法进行聚类。下面是一个使用OPTICS聚类的示例代码:
```python
from sklearn.cluster import OPTICS
import numpy as np
X = np.array(\[\[1, 2\], \[2, 5\], \[3, 6\],\[8, 7\], \[8, 8\], \[7, 3\]\])
clustering = OPTICS(min_samples=2).fit(X)
labels = clustering.labels_
```
在这个示例中,我们使用了scikit-learn库中的OPTICS类进行聚类。首先,我们定义了一个数据集X,然后使用OPTICS算法对数据进行聚类。最后,我们可以通过`labels_`属性获取每个数据点的聚类标签。
总结来说,OPTICS聚类算法是一种基于密度的聚类算法,通过计算可达距离和核心距离来确定数据点的聚类关系。在scikit-learn中,可以使用OPTICS类进行聚类操作。
#### 引用[.reference_title]
- *1* [(4)聚类算法之OPTICS算法](https://blog.csdn.net/LoveCarpenter/article/details/85049135)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down28v1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* *3* [OPTICS聚类算法详解](https://blog.csdn.net/weixin_43569478/article/details/115019317)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down28v1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
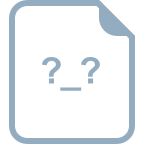
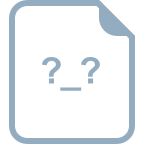
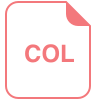
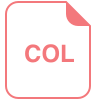
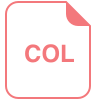
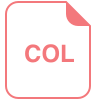
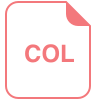






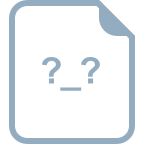
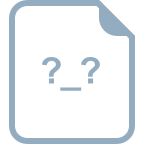