import os import numpy as np from PIL import Image from tensorflow.keras.applications.resnet50 import ResNet50, preprocess_input from sklearn.cluster import DBSCAN from sklearn.manifold import TSNE # 加载 ResNet50 模型 model = ResNet50(weights='imagenet', include_top=False) # 加载并预处理图片,提取特征向量 features = [] for filename in os.listdir('D:/wjd/2'): if filename.endswith('.png'): img = Image.open(os.path.join('D:/wjd/2', filename)) img = img.convert('RGB') # 将 RGBA 转换为 RGB img = img.resize((224, 224)) x = np.array(img) x = np.expand_dims(x, axis=0) x = preprocess_input(x) feature = model.predict(x) feature = np.squeeze(feature) features.append(feature) # 将特征向量进行降维处理 tsne = TSNE(n_components=2) features_tsne = tsne.fit_transform(features) # 将特征向量进行聚类,自动确定聚类的数量 dbscan = DBSCAN(eps=5, min_samples=2).fit(features_tsne) n_clusters = len(set(dbscan.labels_)) # 将每张图片分配到对应的聚类中 for i, label in enumerate(dbscan.labels_): filename = os.listdir('D:/wjd/2')[i] print('{} belongs to cluster {}'.format(filename, label))这段代码出现了AttributeError: 'list' object has no attribute 'shape'这个错误
时间: 2024-03-03 17:50:26 浏览: 127
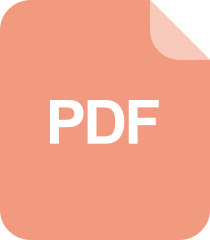
python 3.74 运行import numpy as np 报错lib\site-packages\numpy\__init__.py
这个错误通常发生在尝试对一个列表对象调用 `shape` 属性时。在你的代码中,错误可能是由于 `features` 列表不是 numpy 数组而引起的。`TSNE` 类的 `fit_transform` 方法需要的输入是一个 numpy 数组,因此,将 `features` 列表转换为 numpy 数组可能会解决这个问题。将以下行添加到代码中即可将 `features` 列表转换为 numpy 数组:
```
features = np.array(features)
```
将其添加到 `features` 列表的末尾之后,再次运行代码,应该就不会遇到这个错误了。
阅读全文
相关推荐
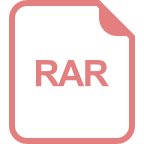





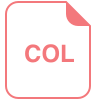
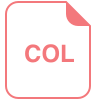
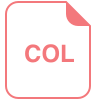







