C++编写一个能对字符串数组由小到大进行排序的小程序
时间: 2024-02-16 11:03:51 浏览: 49
下面是一个简单的C++程序,可以对字符串数组进行由小到大排序:
```cpp
#include <iostream>
#include <algorithm>
#include <string>
using namespace std;
int main()
{
string arr[] = {"apple", "banana", "orange", "grape", "pear"};
int n = sizeof(arr) / sizeof(arr[0]);
sort(arr, arr + n);
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
return 0;
}
```
这个程序使用了 `sort` 函数来对字符串数组进行排序。`sort` 函数需要两个参数,第一个是数组的开始地址,第二个是数组的结束地址(注意不是长度)。在这个例子中,我们使用了 `sizeof` 运算符来获取数组的长度,并将其除以每个元素的长度,从而得到元素的个数。
运行程序后,输出应该是:
```
apple banana grape orange pear
```
这就是按照字典序排序后的结果。
相关问题
用C++编写一个能对字符串数组由小到大进行排序的小程序
以下是使用C++实现字符串数组排序的示例程序:
```c++
#include <iostream>
#include <algorithm>
#include <string>
using namespace std;
int main() {
const int n = 5; // 字符串数组大小
string strs[n] = {"hello", "world", "apple", "orange", "banana"}; // 待排序字符串数组
// 对字符串数组进行排序
sort(strs, strs + n);
// 输出排序后的字符串数组
for (int i = 0; i < n; i++) {
cout << strs[i] << endl;
}
return 0;
}
```
在上面的程序中,我们使用了`sort`函数来对字符串数组进行排序。该函数需要传入两个迭代器表示需要排序的范围,这里我们使用了数组的指针和数组长度来表示。
运行程序后,输出结果为:
```
apple
banana
hello
orange
world
```
可以看到,字符串数组已经按字典序由小到大排好序了。
用c++编写一个能对字符串数组由小到大进行排序的小程序。
以下是使用C++的标准库函数实现字符串数组排序的程序:
```c++
#include <iostream>
#include <algorithm>
#include <cstring>
using namespace std;
int main()
{
const int MAXN = 100;
int n;
string str[MAXN];
cin >> n; // 输入字符串数量
for (int i = 0; i < n; i++)
{
cin >> str[i]; // 输入每个字符串
}
sort(str, str + n); // 使用sort函数进行排序,从str[0]开始,到str[n-1]结束
for (int i = 0; i < n; i++)
{
cout << str[i] << endl; // 输出排序后的字符串
}
return 0;
}
```
此程序中,使用了标准库中的`sort`函数进行排序,`sort`函数的第一个参数是排序的起始位置,第二个参数是排序的结束位置(不包括该位置本身)。在这个例子中,我们将起始位置设置为`str`数组的第一个元素`str[0]`,结束位置设置为`str`数组的最后一个元素`str[n-1]`。
程序中还使用了`string`类型来存储输入的字符串,`string`类型是一个C++标准库中的类型,可以直接使用。`string`类型的排序默认是按照字典序进行排序的,所以使用`sort`函数可以很方便地完成字符串数组的排序。
相关推荐
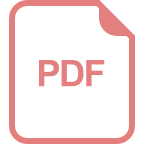
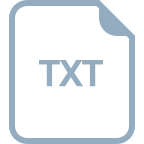
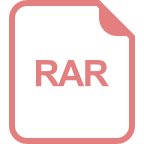













