使用ZipInputStream解压后修改解压的文件夹名称
时间: 2024-04-23 17:27:44 浏览: 23
您可以使用Java的ZipInputStream类来解压文件,并且在解压后修改解压的文件夹名称。下面是示例代码:
```java
import java.io.*;
import java.util.zip.*;
public class ZipExtractor {
public static void extractAndRename(String zipFilePath, String destinationPath, String newFolderName) {
try (ZipInputStream zipIn = new ZipInputStream(new FileInputStream(zipFilePath))) {
ZipEntry entry = zipIn.getNextEntry();
byte[] buffer = new byte[1024];
while (entry != null) {
String filePath = destinationPath + File.separator + entry.getName();
if (!entry.isDirectory()) {
// Extract the file
File outputFile = new File(filePath);
new File(outputFile.getParent()).mkdirs();
try (FileOutputStream fos = new FileOutputStream(outputFile)) {
int len;
while ((len = zipIn.read(buffer)) > 0) {
fos.write(buffer, 0, len);
}
}
} else {
// Rename the folder
String folderPath = destinationPath + File.separator + entry.getName();
String newFolderPath = destinationPath + File.separator + newFolderName;
File folder = new File(folderPath);
File newFolder = new File(newFolderPath);
folder.renameTo(newFolder);
}
zipIn.closeEntry();
entry = zipIn.getNextEntry();
}
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
String zipFilePath = "path/to/your/zip/file.zip";
String destinationPath = "path/to/your/destination/folder";
String newFolderName = "new_folder_name";
extractAndRename(zipFilePath, destinationPath, newFolderName);
}
}
```
您需要将`zipFilePath`设置为要解压的ZIP文件的路径,`destinationPath`设置为要解压到的目标文件夹路径,`newFolderName`设置为要修改的文件夹名称。然后运行`extractAndRename`方法即可完成解压和重命名操作。
请确保您已经在Java环境中设置了正确的文件路径和文件夹名称。
相关推荐
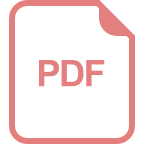
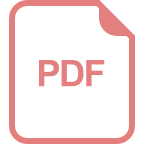
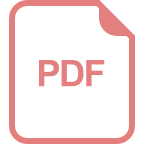














