如何用C语言代码实现文件的读取和写入
时间: 2024-02-28 14:55:39 浏览: 29
在 C 语言中,可以使用标准库中的 `fopen()`、`fread()` 和 `fwrite()` 函数来进行文件的读取和写入操作。以下是一个简单的示例代码:
```c
#include <stdio.h>
int main() {
FILE *inputFile, *outputFile;
char inputFileName[100], outputFileName[100];
char buffer[1024];
int bytesRead;
// 打开输入文件
printf("Enter the name of the input file: ");
scanf("%s", inputFileName);
inputFile = fopen(inputFileName, "rb");
if (inputFile == NULL) {
printf("Unable to open input file!");
return 1;
}
// 打开输出文件
printf("Enter the name of the output file: ");
scanf("%s", outputFileName);
outputFile = fopen(outputFileName, "wb");
if (outputFile == NULL) {
printf("Unable to create output file!");
return 1;
}
// 读取输入文件并写入输出文件
while ((bytesRead = fread(buffer, 1, sizeof(buffer), inputFile)) > 0) {
fwrite(buffer, 1, bytesRead, outputFile);
}
// 关闭文件
fclose(inputFile);
fclose(outputFile);
printf("File copied successfully!");
return 0;
}
```
在这个示例代码中,程序要求用户输入要读取和写入的文件名。然后程序使用 `fopen()` 函数打开输入和输出文件,并检查文件是否打开成功。接着程序使用 `fread()` 函数读取输入文件内容,每次最多读取 `sizeof(buffer)` 个字节,`fread()` 函数返回实际读取的字节数。程序使用 `fwrite()` 函数将读取到的数据写入输出文件。最后程序使用 `fclose()` 函数关闭文件。
请注意,在使用 `fopen()` 函数打开文件时需要指定正确的读取或写入模式。在上面的示例代码中,使用的是 "rb" 和 "wb" 模式,其中 "r" 表示只读模式,"w" 表示只写模式,"b" 表示二进制模式。如果要读取或写入文本文件,应该使用 "r" 或 "w" 模式而不是 "rb" 或 "wb" 模式。
相关推荐
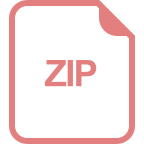













