bresenham算法画圆
时间: 2023-10-12 08:19:38 浏览: 52
Bresenham算法是一种用于计算计算机图形学中的离散点之间的最优路径的算法,常用于画线和画圆等图形的绘制。下面是使用Bresenham算法画圆的步骤:
1. 输入圆心坐标和半径r。
2. 初始化圆心坐标与圆周上的点P0(x0, y0)、P1(x0 + r, y0)、P2(x0, y0 + r)的坐标。
3. 设置初始值d=3-2r。
4. 如果P0在第一象限,则按如下方式移动P1和P2:
- 如果d>0,选择P1和P2中x坐标小的那个点,即y坐标不变,x坐标加1,然后d=d+4(x1-x0)+10。
- 如果d<=0,则选择P1和P2中y坐标大的那个点,即x坐标不变,y坐标加1,然后d=d+4(y1-y0)+6。
5. 绘制P1、P2的对称点,得到第二象限的圆弧。
6. 重复步骤4和5,直到P1和P2相遇。
7. 绘制相遇点的对称点,得到第三象限的圆弧。
8. 重复步骤4、5、7和8,直到P1和P2再次相遇。
9. 绘制相遇点的对称点,得到第四象限的圆弧。
10. 绘制完整的圆形。
下面是使用Python实现Bresenham算法画圆的示例代码:
```python
import matplotlib.pyplot as plt
def draw_circle(center_x, center_y, radius):
x = 0
y = radius
d = 3 - 2 * radius
points = [(center_x, center_y + radius), (center_x + radius, center_y), (center_x, center_y - radius), (center_x - radius, center_y)]
while x <= y:
if d <= 0:
d += 4 * x + 6
else:
d += 4 * (x - y) + 10
y -= 1
x += 1
points.append((center_x + x, center_y + y))
points.append((center_x + x, center_y - y))
points.append((center_x - x, center_y + y))
points.append((center_x - x, center_y - y))
points.append((center_x + y, center_y + x))
points.append((center_x + y, center_y - x))
points.append((center_x - y, center_y + x))
points.append((center_x - y, center_y - x))
for point in points:
plt.plot(point[0], point[1], 'ro')
plt.axis('equal')
plt.show()
draw_circle(0, 0, 5)
```
运行上述代码,就可以在Matplotlib中看到绘制的圆形。
相关推荐
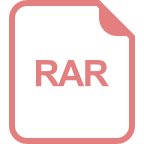














