bresenham算法画圆python
时间: 2023-10-24 12:21:05 浏览: 59
以下是使用bresenham算法在Python中绘制圆的示例代码:
```python
import pygame
# 初始化pygame
pygame.init()
# 设置窗口大小和标题
screen = pygame.display.set_mode((640, 480))
pygame.display.set_caption("Bresenham Circle")
# 设置颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
def draw_circle(xc, yc, x, y):
"""
绘制圆的8个对称点
"""
screen.set_at((xc + x, yc + y), WHITE)
screen.set_at((xc - x, yc + y), WHITE)
screen.set_at((xc + x, yc - y), WHITE)
screen.set_at((xc - x, yc - y), WHITE)
screen.set_at((xc + y, yc + x), WHITE)
screen.set_at((xc - y, yc + x), WHITE)
screen.set_at((xc + y, yc - x), WHITE)
screen.set_at((xc - y, yc - x), WHITE)
def bresenham_circle(xc, yc, r):
"""
Bresenham算法绘制圆
"""
x = 0
y = r
d = 3 - 2 * r
draw_circle(xc, yc, x, y)
while x <= y:
if d < 0:
d = d + 4 * x + 6
else:
d = d + 4 * (x - y) + 10
y = y - 1
x = x + 1
draw_circle(xc, yc, x, y)
# 游戏循环
done = False
while not done:
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
# 填充背景颜色
screen.fill(BLACK)
# 绘制圆
bresenham_circle(320, 240, 100)
# 更新屏幕
pygame.display.flip()
# 退出pygame
pygame.quit()
```
在上述代码中,我们定义了两个函数:`draw_circle()`和`bresenham_circle()`。`draw_circle()`函数用于绘制圆的8个对称点,而`bresenham_circle()`函数使用Bresenham算法绘制圆。
在游戏循环中,我们使用`bresenham_circle()`函数绘制圆,并在屏幕上更新。
你可以根据自己的需求修改代码中的参数,例如圆心坐标和半径。
相关推荐
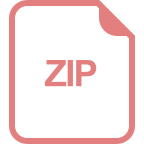
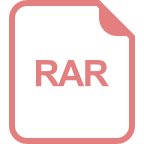










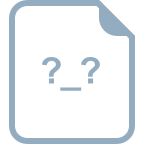