给出Bresenham画圆算法的python代码
时间: 2023-07-23 17:10:22 浏览: 60
下面是 Bresenham 画圆算法的 Python 代码实现:
```python
import pygame
# 初始化 Pygame
pygame.init()
# 定义屏幕宽高
width = 640
height = 480
# 创建窗口
screen = pygame.display.set_mode((width, height))
# 设置窗口标题
pygame.display.set_caption("Bresenham Circle Algorithm")
# 定义圆心坐标和半径
x0 = width // 2
y0 = height // 2
radius = min(width, height) // 4
# 定义 Bresenham 画圆函数
def bresenham_circle(x0, y0, radius):
# 初始化参数
x = 0
y = radius
d = 3 - 2 * radius
# 循环画圆
while x <= y:
# 绘制八个对称点
pygame.draw.circle(screen, (255, 255, 255), (x0 + x, y0 + y), 1)
pygame.draw.circle(screen, (255, 255, 255), (x0 + y, y0 + x), 1)
pygame.draw.circle(screen, (255, 255, 255), (x0 - x, y0 + y), 1)
pygame.draw.circle(screen, (255, 255, 255), (x0 - y, y0 + x), 1)
pygame.draw.circle(screen, (255, 255, 255), (x0 + x, y0 - y), 1)
pygame.draw.circle(screen, (255, 255, 255), (x0 + y, y0 - x), 1)
pygame.draw.circle(screen, (255, 255, 255), (x0 - x, y0 - y), 1)
pygame.draw.circle(screen, (255, 255, 255), (x0 - y, y0 - x), 1)
# 更新参数
if d < 0:
d += 4 * x + 6
else:
d += 4 * (x - y) + 10
y -= 1
x += 1
# 循环绘制圆
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 绘制背景
screen.fill((0, 0, 0))
# 绘制圆
bresenham_circle(x0, y0, radius)
# 更新屏幕
pygame.display.flip()
# 退出 Pygame
pygame.quit()
```
这段代码实现了 Bresenham 画圆算法,并使用 Pygame 库进行图形绘制。与中点画圆算法类似,我们首先定义了圆心坐标和半径,然后定义了 Bresenham 画圆函数。函数中使用 while 循环绘制八个对称点,并根据 Bresenham 画圆算法更新参数。最后,我们使用 Pygame 库循环绘制圆,并更新屏幕。
相关推荐
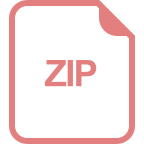
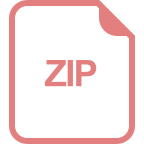










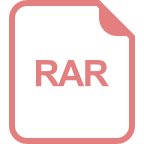
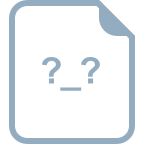