1.编写一个程序用于获取网络中的某个资源(https://www.baidu.com),并将内容显示在命令行窗口中。
时间: 2024-02-09 22:06:57 浏览: 22
好的,您可以使用Python中的requests模块来实现这个问题。以下是示例代码:
```python
import requests
url = 'https://www.baidu.com'
response = requests.get(url)
if response.status_code == 200:
print(response.text)
else:
print('Failed to get the resource.')
```
这个程序会向指定的URL发起一个GET请求,并获取响应的内容,最后将内容打印在命令行窗口中。如果请求成功,状态码为200,就会打印出响应的内容;否则,会提示请求失败。
相关问题
1.编写一个程序用于获取网络中的某个资源(https://www.baidu.com),并将内容显示在命令行窗口中。 用java语言
可以使用Java中的URLConnection类来实现获取网络资源的操作,以下是示例代码:
```java
import java.net.*;
import java.io.*;
public class URLExample {
public static void main(String[] args) {
try {
URL url = new URL("https://www.baidu.com");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
BufferedReader reader = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
运行该程序后,会将百度首页的内容输出到命令行窗口中。需要注意的是,由于网络请求是一个耗时的操作,因此需要在程序中进行异常处理。
用Java编写一个程序用于获取网络中的某个资源(https://www.baidu.com),并将内容显示在命令行窗口中。
好的,您可以使用Java中的HttpURLConnection类和BufferedReader类来实现这个问题。以下是示例代码:
```java
import java.net.HttpURLConnection;
import java.net.URL;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class Main {
public static void main(String[] args) throws Exception {
String url = "https://www.baidu.com";
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
// 设置请求方法为GET
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// 打印响应内容
System.out.println(response.toString());
} else {
System.out.println("Failed to get the resource.");
}
}
}
```
这个程序会向指定的URL发起一个GET请求,并获取响应的内容,最后将内容打印在命令行窗口中。如果请求成功,状态码为200,就会打印出响应的内容;否则,会提示请求失败。
相关推荐
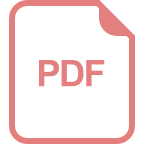
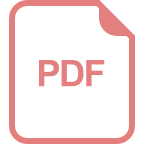
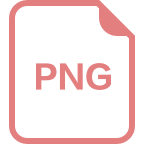












