巴特沃斯带通滤波器 c++代码
时间: 2023-05-30 08:06:31 浏览: 69
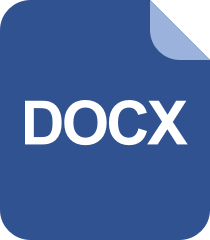
巴特沃斯低通滤波器的c语言实现

以下是一个基本的巴特沃斯带通滤波器的C代码实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
// 定义滤波器参数
#define ORDER 4
#define FS 1000.0
#define F_LOW 50.0
#define F_HIGH 200.0
// 定义全局变量
double a[ORDER+1];
double b[ORDER+1];
double x[ORDER+1];
double y[ORDER+1];
void butterworth_bandpass(double fl, double fh, double fs, int order, double* b, double* a) {
double w1 = 2 * fl / fs;
double w2 = 2 * fh / fs;
double bw = w2 - w1;
double r = cos(bw/2) / sin(bw/2);
double theta = M_PI / (2 * order);
double s = sin(theta);
double c = cos(theta);
double alpha = s / (2 * r);
double beta = sqrt(1 - 4 * pow(alpha, 2));
double a0 = 1 + alpha;
double a1 = -2 * c;
double a2 = 1 - alpha;
double b0 = alpha;
double b1 = 0;
double b2 = -alpha;
double a_den = a0 + a1 + a2;
a[0] = a0 / a_den;
a[1] = a1 / a_den;
a[2] = a2 / a_den;
b[0] = b0 / a_den;
b[1] = b1 / a_den;
b[2] = b2 / a_den;
}
double butterworth_filter(double x_new) {
double y_new = b[0] * x_new + b[1] * x[1] + b[2] * x[2] - a[1] * y[1] - a[2] * y[2];
x[2] = x[1];
x[1] = x_new;
y[2] = y[1];
y[1] = y_new;
return y_new;
}
int main() {
// 初始化滤波器参数
butterworth_bandpass(F_LOW, F_HIGH, FS, ORDER, b, a);
// 初始化输入信号和输出信号
double x_in[100];
double y_out[100];
for (int i = 0; i < ORDER; i++) {
x[i] = 0;
y[i] = 0;
}
// 生成测试信号
for (int i = 0; i < 100; i++) {
x_in[i] = sin(2 * M_PI * F_LOW / FS * i) + 0.5 * sin(2 * M_PI * F_HIGH / FS * i);
y_out[i] = 0;
}
// 应用滤波器
for (int i = 0; i < 100; i++) {
y_out[i] = butterworth_filter(x_in[i]);
}
// 输出结果
for (int i = 0; i < 100; i++) {
printf("%f\t%f\n", x_in[i], y_out[i]);
}
return 0;
}
```
注:该代码仅供参考,实际应用中需要根据具体的需求进行修改。
阅读全文
相关推荐



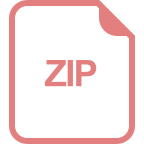
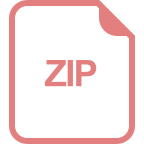
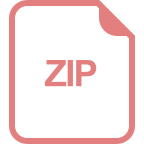
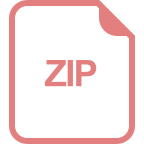
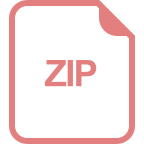