设计图形Shape类及其子类圆Circle和矩形Rectangle类。图形Shape类中包括位置坐标(x,y),圆类Circle新增半径r,矩形Rectangle类新增宽w和高h,编写三个类的构造方法实现成员变量初始化。
时间: 2024-05-10 10:18:24 浏览: 109
以下是示例代码:
```python
class Shape:
def __init__(self, x, y):
self.x = x
self.y = y
class Circle(Shape):
def __init__(self, x, y, r):
super().__init__(x, y)
self.r = r
class Rectangle(Shape):
def __init__(self, x, y, w, h):
super().__init__(x, y)
self.w = w
self.h = h
```
在这个示例中,我们使用了继承来创建 Circle 和 Rectangle 类,这两个类都从 Shape 类继承。在 Shape 类中,我们定义了位置坐标 x 和 y,而在 Circle 类中,我们新增了半径 r,而在 Rectangle 类中,我们新增了宽 w 和高 h。每个类的构造函数都调用了超类(即 Shape)的构造函数,并将所需的参数传递给它。这样,我们就可以通过创建这些类的实例来初始化它们的成员变量。
相关问题
设计图形Shape接口及其子类圆Circle和矩形Rectangle类。接口中声明求图形面积的方法getArea,并两个子类中实现。再定义测试类Test,实现圆和矩形面积的输出。(接口)
下面是Java代码实现:
```
// Shape接口
public interface Shape {
double getArea();
}
// 圆类
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
}
// 矩形类
public class Rectangle implements Shape {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
public double getLength() {
return length;
}
public void setLength(double length) {
this.length = length;
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
this.width = width;
}
@Override
public double getArea() {
return length * width;
}
}
// 测试类
public class Test {
public static void main(String[] args) {
Circle c = new Circle(5);
System.out.println("圆的面积为:" + c.getArea());
Rectangle r = new Rectangle(3, 4);
System.out.println("矩形的面积为:" + r.getArea());
}
}
```
java设计图形shape类及其子类Circle和Rectangle
好的,我理解了您的要求。下面是 Java 中的 Shape 类及其子类 Circle 和 Rectangle 的设计示例:
```java
/* Shape 类 */
public abstract class Shape {
protected int x;
protected int y;
public Shape(int x, int y) {
this.x = x;
this.y = y;
}
public abstract double area();
}
/* Circle 类 */
public class Circle extends Shape {
private int radius;
public Circle(int x, int y, int radius) {
super(x, y);
this.radius = radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
}
/* Rectangle 类 */
public class Rectangle extends Shape {
private int width;
private int height;
public Rectangle(int x, int y, int width, int height) {
super(x, y);
this.width = width;
this.height = height;
}
@Override
public double area() {
return width * height;
}
}
```
在这个示例中,Shape 类是一个抽象类,它有两个属性 x 和 y,表示图形的位置。它还有一个抽象方法 area(),表示计算图形的面积。
Circle 类继承自 Shape 类,它有一个额外的属性 radius,表示圆的半径。Circle 类实现了 Shape 类的 area() 方法,用于计算圆的面积。
Rectangle 类也继承自 Shape 类,它有两个额外的属性 width 和 height,表示矩形的宽和高。Rectangle 类同样实现了 Shape 类的 area() 方法,用于计算矩形的面积。
阅读全文
相关推荐
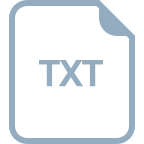
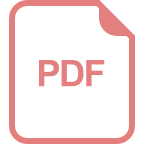
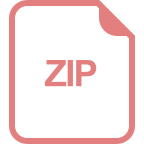












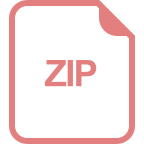
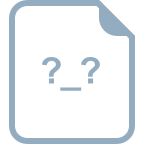