用java编写程序,统计文件中单词出现次数,单词间以空格,tab或回车间隔。
时间: 2023-08-12 22:08:48 浏览: 50
可以使用Java中的HashMap来统计单词出现的次数,具体实现如下:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.HashMap;
public class WordCount {
public static void main(String[] args) {
HashMap<String, Integer> wordCountMap = new HashMap<>();
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
String[] words = line.split("[ \t\n]+");
for (String word : words) {
if (!wordCountMap.containsKey(word)) {
wordCountMap.put(word, 1);
} else {
wordCountMap.put(word, wordCountMap.get(word) + 1);
}
}
}
} catch (IOException e) {
e.printStackTrace();
}
for (String word : wordCountMap.keySet()) {
System.out.println(word + " : " + wordCountMap.get(word));
}
}
}
```
上述代码中,首先创建了一个HashMap对象用于存储单词出现的次数。然后使用BufferedReader读取文件,逐行读取文件内容,并使用split方法将每行内容分割成单词,使用正则表达式"[ \t\n]+"来表示单词之间可以通过空格、tab或回车进行分割。然后遍历每个单词,如果单词在HashMap中不存在,则将其加入HashMap并将出现次数设置为1;否则,将出现次数加1。最后,遍历HashMap并输出每个单词出现的次数。
相关推荐
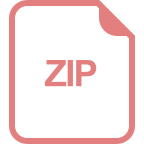














