用tensorflow写LSTM模型预测各年龄死亡率代码
时间: 2023-11-14 22:28:42 浏览: 42
以下是一个使用TensorFlow实现LSTM模型进行各年龄死亡率预测的代码示例:
```python
import tensorflow as tf
import numpy as np
import pandas as pd
# 加载数据
data = pd.read_csv('data.csv')
x = data.iloc[:, :-1].values
y = data.iloc[:, -1].values
# 数据预处理
x = np.reshape(x, (x.shape[0], x.shape[1], 1))
# 划分训练集和测试集
train_size = int(len(x) * 0.7)
test_size = len(x) - train_size
train_x, test_x = x[0:train_size,:], x[train_size:len(x),:]
train_y, test_y = y[0:train_size], y[train_size:len(y)]
# 构建LSTM模型
model = tf.keras.Sequential()
model.add(tf.keras.layers.LSTM(50, input_shape=(train_x.shape[1], train_x.shape[2])))
model.add(tf.keras.layers.Dense(1))
model.compile(loss='mean_squared_error', optimizer='adam')
# 训练模型
model.fit(train_x, train_y, epochs=100, batch_size=64, validation_data=(test_x, test_y), verbose=2, shuffle=False)
# 预测
train_predict = model.predict(train_x)
test_predict = model.predict(test_x)
# 可视化预测结果
import matplotlib.pyplot as plt
plt.plot(train_y)
plt.plot(train_predict)
plt.plot(test_y)
plt.plot(test_predict)
plt.show()
```
其中,`data.csv`是包含历史死亡率数据的CSV文件。代码使用了`pandas`库来读取和处理数据,使用了`numpy`库来进行数据预处理和划分训练集和测试集。LSTM模型使用了`tensorflow`库中的`tf.keras.layers.LSTM`和`tf.keras.layers.Dense`来构建。模型的训练使用了`model.fit()`方法,预测使用了`model.predict()`方法。最后,代码使用`matplotlib`库将预测结果可视化。
相关推荐
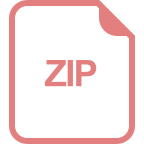
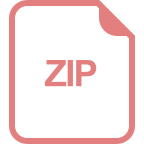














