用tkinter写一个汇率转换
时间: 2023-12-09 08:26:07 浏览: 139
好的,下面是一个简单的汇率转换器的示例代码,使用 Python 的 tkinter 模块实现 GUI:
```python
import tkinter as tk
import requests
class CurrencyConverter:
def __init__(self, url):
self.data = requests.get(url).json()
self.currencies = self.data['rates']
def convert(self, from_currency, to_currency, amount):
initial_amount = amount
if from_currency != 'USD':
amount = amount / self.currencies[from_currency]
# limiting the precision to 4 decimal places
amount = round(amount * self.currencies[to_currency], 4)
return amount
class App:
def __init__(self, master):
self.master = master
self.converter = CurrencyConverter('https://api.exchangerate-api.com/v4/latest/USD')
master.title("Currency Converter")
# create dropdown boxes for currencies
self.from_currency = tk.StringVar(master)
self.to_currency = tk.StringVar(master)
from_currency_dropdown = tk.OptionMenu(master, self.from_currency, *self.converter.currencies.keys())
to_currency_dropdown = tk.OptionMenu(master, self.to_currency, *self.converter.currencies.keys())
from_currency_dropdown.grid(row=0, column=0, padx=5, pady=5)
to_currency_dropdown.grid(row=0, column=1, padx=5, pady=5)
# create entry widget for amount
self.amount = tk.Entry(master)
self.amount.grid(row=1, column=0, padx=5, pady=5)
# create button to perform conversion
self.convert_button = tk.Button(master, text="Convert", command=self.perform_conversion)
self.convert_button.grid(row=1, column=1, padx=5, pady=5)
# create label to display converted amount
self.converted_amount = tk.Label(master, text="")
self.converted_amount.grid(row=2, column=0, columnspan=2, padx=5, pady=5)
def perform_conversion(self):
amount = float(self.amount.get())
from_currency = self.from_currency.get()
to_currency = self.to_currency.get()
converted_amount = self.converter.convert(from_currency, to_currency, amount)
self.converted_amount.config(text=str(converted_amount))
root = tk.Tk()
app = App(root)
root.mainloop()
```
这个程序使用 exchangerate-api.com 提供的 API 获取货币汇率数据,然后在 GUI 中提供下拉列表、输入框和按钮,让用户输入转换前的货币种类、转换后的货币种类以及转换金额,最后显示转换后的金额。
阅读全文
相关推荐
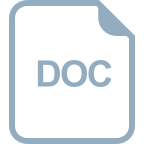
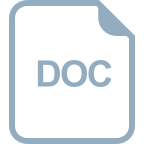
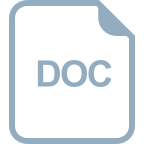
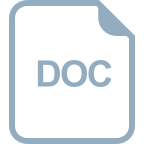
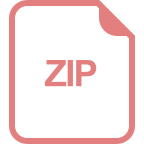
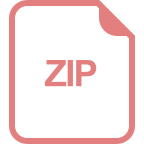
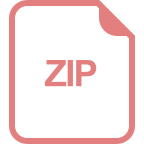
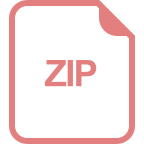
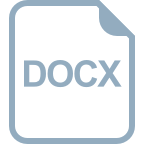
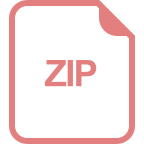
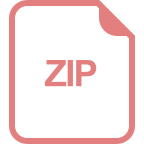
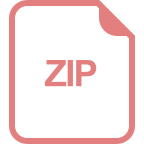
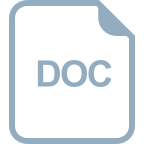


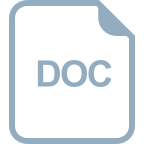
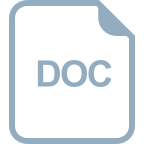
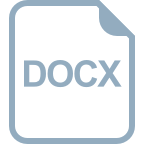