vue3 <script setup>通过ref修改子组件的数组数据
时间: 2023-11-28 17:50:33 浏览: 34
可以使用`toRef`和`toRefs`来将子组件的数组数据转换为响应式的对象,然后通过修改这个对象来修改子组件的数组数据。
例如,假设子组件中有一个名为`list`的数组数据,可以在父组件中这样使用`toRef`来将其转换为响应式的对象:
```javascript
import { toRef } from 'vue'
setup() {
const childComponentRef = ref(null)
const listRef = toRef(childComponentRef.value, 'list')
const modifyList = () => {
listRef.value.push('new item')
}
return {
childComponentRef,
modifyList
}
}
```
然后在模板中,可以将子组件的`ref`属性绑定到`childComponentRef`上,并在需要的地方调用`modifyList`方法来修改子组件的数组数据:
```html
<template>
<child-component ref="childComponentRef" />
<button @click="modifyList">修改子组件数组数据</button>
</template>
```
相关问题
vue3通过ref修改子组件的数组数据
可以通过在子组件中使用 ref,然后在父组件中通过 ref 获取子组件实例,并调用子组件的方法来修改子组件的数组数据。
例如,假设子组件名为 Child,数组数据名为 list,可以如下定义子组件:
```
<template>
<div>
<div v-for="(item, index) in list" :key="index">
{{ item }}
</div>
</div>
</template>
<script>
export default {
name: 'Child',
props: {
list: { type: Array }
}
}
</script>
```
在父组件中,可以使用 ref 获取子组件实例,并调用子组件的方法来修改子组件的数组数据:
```
<template>
<div>
<button @click="changeList">修改子组件数组</button>
<Child ref="child" :list="list"></Child>
</div>
</template>
<script>
import { ref } from 'vue'
import Child from './Child.vue'
export default {
name: 'Parent',
components: { Child },
setup() {
const list = ref(['A', 'B', 'C'])
const changeList = () => {
const child = this.$refs.child
if (child) {
child.list[0] = 'D'
child.list[1] = 'E'
child.list[2] = 'F'
}
}
return { list, changeList }
}
}
</script>
```
在修改子组件的数组数据时,需要注意的是,直接修改子组件的数组数据可能会导致 Vue 的响应式系统失效,因此最好使用 Vue 提供的 API 来修改数据,例如使用 `splice` 方法来替换数组元素。
<script setup>用这个方法写一遍
好的,下面是使用 `<script setup>` 写法的示例代码,来实现父组件中的数组传递,并在子组件中进行修改:
父组件:
```html
<template>
<div>
<input v-for="(item, index) in list" :key="index" v-model="list[index]" />
<ChildComponent :list="list" @update:list="list = $event" />
</div>
</template>
<script setup>
import { ref } from "vue";
import ChildComponent from "./ChildComponent.vue";
const list = ref(["apple", "banana", "orange"]);
export default {
components: {
ChildComponent,
},
};
</script>
```
在上面的代码中,我们使用了 `<script setup>` 语法糖来定义了一个响应式变量 `list`,并将其作为 prop 传递给了子组件 `ChildComponent`。
子组件:
```html
<template>
<div>
<input v-for="(item, index) in list" :key="index" v-model="list[index]" @input="updateList" />
</div>
</template>
<script setup>
import { ref, watch, emit } from "vue";
const props = defineProps({
list: {
type: Array,
required: true,
},
});
const list = ref(props.list);
const updateList = () => {
emit("update:list", list.value);
};
watch(props, () => {
list.value = props.list;
});
export default {
props,
list,
updateList,
};
</script>
```
在上面的代码中,我们首先使用 `defineProps` 函数来定义了一个 prop `list`,然后使用 `ref` 函数定义了一个响应式变量 `list` 来接收这个数组。
接着,我们定义了一个方法 `updateList`,用于在 input 中修改 `list` 的值,并将其通过 `emit` 函数传递回父组件。
最后,我们使用 `watch` 函数来监听父组件传递的 `list` 的变化,并更新子组件中的 `list` 值。
这样,我们就可以使用 `<script setup>` 写法来实现父组件中的数组传递,并在子组件中进行修改。需要注意的是,`<script setup>` 写法需要使用 `defineProps` 函数来定义 props,使用 `emit` 函数来触发事件,以及使用 `watch` 函数来监听 props 的变化。
相关推荐
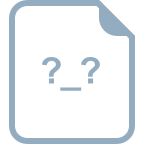
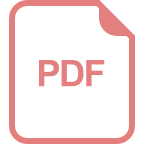












