defaultdict(list)写一段代码python
时间: 2024-02-02 10:03:02 浏览: 21
好的,以下是一个使用 `defaultdict(list)` 的示例代码:
```
from collections import defaultdict
# 创建一个 defaultdict 对象,其默认值为一个空列表
d = defaultdict(list)
# 向字典中添加一些数据
d['fruits'].append('apple')
d['fruits'].append('banana')
d['fruits'].append('orange')
d['vegetables'].append('carrot')
d['vegetables'].append('broccoli')
# 打印字典中的数据
print(d)
```
输出:
```
defaultdict(<class 'list'>, {'fruits': ['apple', 'banana', 'orange'], 'vegetables': ['carrot', 'broccoli']})
```
在这个例子中,我们创建了一个 `defaultdict(list)` 对象 `d`,其默认值为一个空列表。然后,我们向字典中添加了一些数据,其中键为 `fruits` 和 `vegetables`,对应的值为一个列表。由于字典的默认值为一个空列表,因此我们可以直接使用 `append` 方法向列表中添加元素,而不需要先进行键的检查。最后,我们打印了整个字典,其中包含了所有添加的数据。
相关问题
python写一段目标检测代码
以下是一段使用 TensorFlow Object Detection API 进行目标检测的 Python 代码:
```python
import numpy as np
import os
import six.moves.urllib as urllib
import sys
import tarfile
import tensorflow as tf
import zipfile
from collections import defaultdict
from io import StringIO
from matplotlib import pyplot as plt
from PIL import Image
# This is needed since the notebook is stored in the object_detection folder.
sys.path.append("..")
# Import utilites
from utils import label_map_util
from utils import visualization_utils as vis_util
# Name of the directory containing the object detection module we're using
MODEL_NAME = 'ssd_mobilenet_v1_coco_2017_11_17'
# Path to frozen detection graph. This is the actual model that is used for the object detection.
PATH_TO_CKPT = MODEL_NAME + '/frozen_inference_graph.pb'
# List of the strings that is used to add correct label for each box.
PATH_TO_LABELS = os.path.join('data', 'mscoco_label_map.pbtxt')
NUM_CLASSES = 90
# Load the label map.
label_map = label_map_util.load_labelmap(PATH_TO_LABELS)
categories = label_map_util.convert_label_map_to_categories(label_map, max_num_classes=NUM_CLASSES, use_display_name=True)
category_index = label_map_util.create_category_index(categories)
# Load the Tensorflow model into memory.
detection_graph = tf.Graph()
with detection_graph.as_default():
od_graph_def = tf.GraphDef()
with tf.gfile.GFile(PATH_TO_CKPT, 'rb') as fid:
serialized_graph = fid.read()
od_graph_def.ParseFromString(serialized_graph)
tf.import_graph_def(od_graph_def, name='')
sess = tf.Session(graph=detection_graph)
# Define input and output tensors (i.e. data) for the object detection classifier
# Input tensor is the image
image_tensor = detection_graph.get_tensor_by_name('image_tensor:0')
# Output tensors are the detection boxes, scores, and classes
# Each box represents a part of the image where a particular object was detected
detection_boxes = detection_graph.get_tensor_by_name('detection_boxes:0')
# Each score represents level of confidence for each of the objects.
# The score is shown on the result image, together with the class label.
detection_scores = detection_graph.get_tensor_by_name('detection_scores:0')
detection_classes = detection_graph.get_tensor_by_name('detection_classes:0')
# Number of objects detected
num_detections = detection_graph.get_tensor_by_name('num_detections:0')
# Load image using OpenCV and
# expand image dimensions to have shape: [1, None, None, 3]
# i.e. a single-column array, where each item in the column has the pixel RGB value
image = cv2.imread('image.jpg')
image_expanded = np.expand_dims(image, axis=0)
# Perform the actual detection by running the model with the image as input
(boxes, scores, classes, num) = sess.run(
[detection_boxes, detection_scores, detection_classes, num_detections],
feed_dict={image_tensor: image_expanded})
# Draw the results of the detection (aka 'visulaize the results')
vis_util.visualize_boxes_and_labels_on_image_array(
image,
np.squeeze(boxes),
np.squeeze(classes).astype(np.int32),
np.squeeze(scores),
category_index,
use_normalized_coordinates=True,
line_thickness=8,
min_score_thresh=0.60)
# All the results have been drawn on image. Now display the image.
cv2.imshow('Object detector', image)
# Press any key to close the image
cv2.waitKey(0)
# Clean up
cv2.destroyAllWindows()
```
帮我写一段最小生成树的代码
当然可以!以下是一个基于 Prim 算法的最小生成树(Minimum Spanning Tree)的代码示例:
```python
from collections import defaultdict
def prim(graph):
# 存储最小生成树的边
mst = []
# 存储顶点是否已经被访问的状态
visited = defaultdict(bool)
# 选择一个起始顶点
start_vertex = list(graph.keys())[0]
visited[start_vertex] = True
while len(mst) < len(graph) - 1:
min_weight = float('inf')
min_edge = None
# 遍历已访问的顶点,寻找权值最小的边
for vertex in graph:
if visited[vertex]:
for neighbor, weight in graph[vertex]:
if not visited[neighbor] and weight < min_weight:
min_weight = weight
min_edge = (vertex, neighbor)
# 将找到的最小边加入最小生成树,标记顶点为已访问
if min_edge:
mst.append(min_edge)
visited[min_edge[1]] = True
return mst
# 测试
graph = {
'A': [('B', 2), ('C', 3)],
'B': [('A', 2), ('C', 4), ('D', 3)],
'C': [('A', 3), ('B', 4), ('D', 5)],
'D': [('B', 3), ('C', 5)]
}
minimum_spanning_tree = prim(graph)
for edge in minimum_spanning_tree:
print(edge)
```
这段代码使用了图的邻接表来表示图,`graph` 是一个字典,键表示顶点,值是一个列表,每个元素是一个二元组,表示与该顶点相邻的顶点及其边的权值。`prim()` 函数使用 Prim 算法来生成最小生成树,并返回最小生成树的边。
请注意,这只是一个示例代码,你可以根据自己的需求进行修改和扩展。希望对你有帮助!
相关推荐
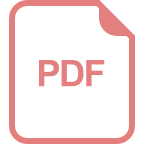
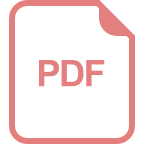












