通过sokect来接收外部的数据包,设计缓存类来管理这部分数据包的C++代码
时间: 2023-05-15 12:07:30 浏览: 113
非常感谢您的提问!以下是一个简单的示例代码,用于通过socket接收外部数据包,并使用缓存类来管理这些数据包:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#define MAX_BUFFER_SIZE 1024
// 缓存类
typedef struct {
char* buffer;
int size;
int capacity;
} Cache;
// 初始化缓存类
void init_cache(Cache* cache) {
cache->buffer = (char*)malloc(MAX_BUFFER_SIZE);
cache->size = 0;
cache->capacity = MAX_BUFFER_SIZE;
}
// 释放缓存类
void free_cache(Cache* cache) {
free(cache->buffer);
cache->buffer = NULL;
cache->size = 0;
cache->capacity = 0;
}
// 扩展缓存类
void expand_cache(Cache* cache, int size) {
if (cache->size + size > cache->capacity) {
int new_capacity = cache->capacity * 2;
while (new_capacity < cache->size + size) {
new_capacity *= 2;
}
char* new_buffer = (char*)malloc(new_capacity);
memcpy(new_buffer, cache->buffer, cache->size);
free(cache->buffer);
cache->buffer = new_buffer;
cache->capacity = new_capacity;
}
}
// 接收数据包
int receive_packet(int sockfd, Cache* cache) {
char buffer[MAX_BUFFER_SIZE];
int n = recv(sockfd, buffer, MAX_BUFFER_SIZE, 0);
if (n <= 0) {
return n;
}
expand_cache(cache, n);
memcpy(cache->buffer + cache->size, buffer, n);
cache->size += n;
return n;
}
int main(int argc, char* argv[]) {
if (argc != 2) {
printf("Usage: %s <port>\n", argv[0]);
return 1;
}
int port = atoi(argv[1]);
// 创建socket
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd < 0) {
perror("socket");
return 1;
}
// 绑定端口
struct sockaddr_in addr;
memset(&addr, 0, sizeof(addr));
addr.sin_family = AF_INET;
addr.sin_addr.s_addr = htonl(INADDR_ANY);
addr.sin_port = htons(port);
if (bind(sockfd, (struct sockaddr*)&addr, sizeof(addr)) < 0) {
perror("bind");
return 1;
}
// 监听端口
if (listen(sockfd, 5) < 0) {
perror("listen");
return 1;
}
printf("Listening on port %d...\n", port);
// 初始化缓存类
Cache cache;
init_cache(&cache);
while (1) {
// 接受连接
struct sockaddr_in client_addr;
socklen_t client_addrlen = sizeof(client_addr);
int client_sockfd = accept(sockfd, (struct sockaddr*)&client_addr, &client_addrlen);
if (client_sockfd < 0) {
perror("accept");
continue;
}
printf("Accepted connection from %s:%d\n", inet_ntoa(client_addr.sin_addr), ntohs(client_addr.sin_port));
// 接收数据包
while (1) {
int n = receive_packet(client_sockfd, &cache);
if (n <= 0) {
if (n < 0) {
perror("recv");
}
break;
}
}
// 关闭连接
close(client_sockfd);
printf("Closed connection from %s:%d\n", inet_ntoa(client_addr.sin_addr), ntohs(client_addr.sin_port));
// 清空缓存
free_cache(&cache);
init_cache(&cache);
}
// 关闭socket
close(sockfd);
return 0;
}
```
希望这个示例代码能够帮助您解决问题!如果您还有其它问题,请随时提出,我会尽力回答。
阅读全文
相关推荐









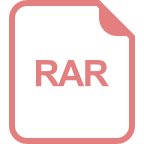

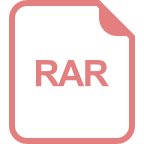



