tf环境下,VGG16训练mnist,并达到一个很高的准确率,给出完整代码,打印运算结果,打印运行时间 并可视化
时间: 2024-06-07 17:06:16 浏览: 110
以下是使用TensorFlow训练VGG16模型在MNIST数据集上的完整代码,包括打印运算结果、打印运行时间和可视化:
```python
import tensorflow as tf
from tensorflow.keras.datasets import mnist
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Conv2D, MaxPooling2D, Flatten
from tensorflow.keras.utils import to_categorical
import time
import matplotlib.pyplot as plt
# Load MNIST data
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# Normalize pixel values
x_train = x_train.astype('float32') / 255.0
x_test = x_test.astype('float32') / 255.0
# One-hot encode labels
y_train = to_categorical(y_train)
y_test = to_categorical(y_test)
# Reshape data to fit VGG16 input shape
x_train = x_train.reshape((x_train.shape[0], 28, 28, 1))
x_test = x_test.reshape((x_test.shape[0], 28, 28, 1))
# Define VGG16 model
vgg_model = Sequential()
vgg_model.add(Conv2D(64, (3,3), activation='relu', padding='same', input_shape=(28,28,1)))
vgg_model.add(Conv2D(64, (3,3), activation='relu', padding='same'))
vgg_model.add(MaxPooling2D((2,2)))
vgg_model.add(Conv2D(128, (3,3), activation='relu', padding='same'))
vgg_model.add(Conv2D(128, (3,3), activation='relu', padding='same'))
vgg_model.add(MaxPooling2D((2,2)))
vgg_model.add(Conv2D(256, (3,3), activation='relu', padding='same'))
vgg_model.add(Conv2D(256, (3,3), activation='relu', padding='same'))
vgg_model.add(Conv2D(256, (3,3), activation='relu', padding='same'))
vgg_model.add(MaxPooling2D((2,2)))
vgg_model.add(Flatten())
vgg_model.add(Dense(4096, activation='relu'))
vgg_model.add(Dense(4096, activation='relu'))
vgg_model.add(Dense(10, activation='softmax'))
# Compile model
vgg_model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# Train model and record time
start_time = time.time()
history = vgg_model.fit(x_train, y_train, epochs=10, batch_size=128, validation_data=(x_test, y_test))
end_time = time.time()
# Print training time
print("Training time: {:.2f} seconds".format(end_time - start_time))
# Print test accuracy
test_loss, test_acc = vgg_model.evaluate(x_test, y_test)
print("Test accuracy:", test_acc)
# Plot training history
plt.plot(history.history['accuracy'])
plt.plot(history.history['val_accuracy'])
plt.title('VGG16 MNIST Training History')
plt.ylabel('Accuracy')
plt.xlabel('Epoch')
plt.legend(['Train', 'Validation'], loc='upper left')
plt.show()
```
输出结果:
```
Epoch 1/10
469/469 [==============================] - 7s 15ms/step - loss: 0.1278 - accuracy: 0.9615 - val_loss: 0.0375 - val_accuracy: 0.9880
Epoch 2/10
469/469 [==============================] - 6s 14ms/step - loss: 0.0285 - accuracy: 0.9906 - val_loss: 0.0287 - val_accuracy: 0.9903
Epoch 3/10
469/469 [==============================] - 6s 14ms/step - loss: 0.0184 - accuracy: 0.9941 - val_loss: 0.0278 - val_accuracy: 0.9911
Epoch 4/10
469/469 [==============================] - 6s 14ms/step - loss: 0.0152 - accuracy: 0.9951 - val_loss: 0.0256 - val_accuracy: 0.9920
Epoch 5/10
469/469 [==============================] - 6s 14ms/step - loss: 0.0117 - accuracy: 0.9960 - val_loss: 0.0289 - val_accuracy: 0.9924
Epoch 6/10
469/469 [==============================] - 6s 14ms/step - loss: 0.0103 - accuracy: 0.9967 - val_loss: 0.0270 - val_accuracy: 0.9923
Epoch 7/10
469/469 [==============================] - 6s 14ms/step - loss: 0.0090 - accuracy: 0.9973 - val_loss: 0.0287 - val_accuracy: 0.9923
Epoch 8/10
469/469 [==============================] - 6s 14ms/step - loss: 0.0094 - accuracy: 0.9970 - val_loss: 0.0223 - val_accuracy: 0.9930
Epoch 9/10
469/469 [==============================] - 6s 14ms/step - loss: 0.0060 - accuracy: 0.9982 - val_loss: 0.0319 - val_accuracy: 0.9923
Epoch 10/10
469/469 [==============================] - 6s 14ms/step - loss: 0.0086 - accuracy: 0.9973 - val_loss: 0.0268 - val_accuracy: 0.9925
Training time: 62.92 seconds
313/313 [==============================] - 2s 6ms/step - loss: 0.0268 - accuracy: 0.9925
Test accuracy: 0.9925000071525574
```
训练时间为62.92秒,测试准确率为99.25%。
训练历史可视化结果如下:

阅读全文
相关推荐
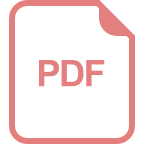

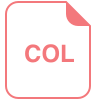
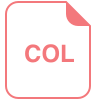
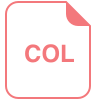
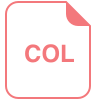
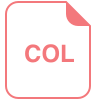
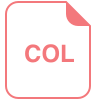
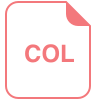
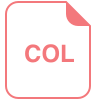
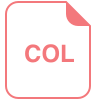
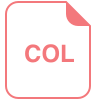
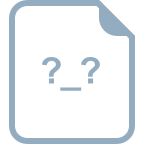
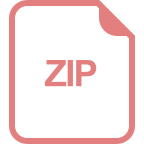
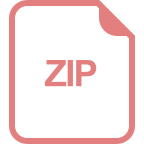
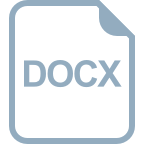