用c语言写一段可以玩的贪吃蛇的代码
时间: 2023-06-06 17:06:21 浏览: 85
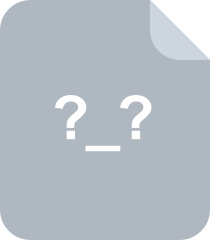
用C语言写的贪吃蛇游戏代码
Sure, here is a simple C program for a playable Snake game:
```
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <time.h>
#define HEIGHT 20
#define WIDTH 30
int map[HEIGHT][WIDTH];
int snake[HEIGHT*WIDTH][2];
int len, score;
int dire, over;
char key;
clock_t last;
void init(){
int i, j;
len = 3;
score = 0;
dire = 2;
over = 0;
for(i=0;i<HEIGHT;i++){
for(j=0;j<WIDTH;j++){
if(i==0 || i==HEIGHT-1 || j==0 || j==WIDTH-1)
map[i][j] = -1;
else
map[i][j] = 0;
}
}
for(i=0;i<len;i++){
snake[i][0] = HEIGHT/2;
snake[i][1] = i+2;
map[snake[i][0]][snake[i][1]] = 1;
}
snake[0][1]++;
map[snake[0][0]][snake[0][1]] = 2;
srand(time(NULL));
}
void print(){
system("cls");
int i, j;
for(i=0;i<HEIGHT;i++){
for(j=0;j<WIDTH;j++){
switch(map[i][j]){
case 0: printf(" "); break;
case -1: printf("#"); break;
case 1: printf("*"); break;
case 2: printf("@"); break;
case 3: printf("$"); break;
}
}
printf("\n");
}
printf("Score: %d\n", score);
}
void update(){
int i, j;
for(i=len-1;i>0;i--){
snake[i][0] = snake[i-1][0];
snake[i][1] = snake[i-1][1];
}
switch(dire){
case 0: snake[0][0]--; break;
case 1: snake[0][1]++; break;
case 2: snake[0][0]++; break;
case 3: snake[0][1]--; break;
}
if(map[snake[0][0]][snake[0][1]]==1)
over = 1;
if(map[snake[0][0]][snake[0][1]]==-1)
over = 1;
if(map[snake[0][0]][snake[0][1]]==3){
score++;
len++;
map[rand()%(HEIGHT-2)+1][rand()%(WIDTH-2)+1] = 3;
}
map[snake[0][0]][snake[0][1]] = 2;
map[snake[len-1][0]][snake[len-1][1]] = 0;
}
void input(){
char tmp;
if(kbhit()){
key = getch();
if(key=='w' && dire!=2) dire = 0;
if(key=='d' && dire!=3) dire = 1;
if(key=='s' && dire!=0) dire = 2;
if(key=='a' && dire!=1) dire = 3;
}
}
int main(){
init();
while(!over){
print();
input();
if(clock()-last>200){
last = clock();
update();
}
}
printf("Game over! Your score is %d\n", score);
return 0;
}
```
注意:此程序依赖于 <conio.h> 和 time.h 库,应在windows平台上编译和运行。
阅读全文
相关推荐






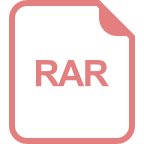
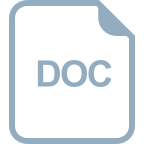







