引用 #include <string> “std::getline”: 未找到匹配的重载函数
时间: 2023-08-06 08:09:11 浏览: 647
如果你在使用 `std::getline` 函数时遇到了“std::getline: 未找到匹配的重载函数”的错误,并且已经包含了 `<string>` 头文件,那么可能是因为你的编译器不支持 C++11 标准或以上的标准。在 C++11 标准中,`std::getline` 函数有一个重载形式,可以接受一个右值引用作为第二个参数,如下所示:
```c++
std::getline(std::istream&& input, std::string& str, char delim);
```
如果你的编译器不支持这个重载形式,你可以使用另一种形式,将第二个参数改为一个普通的引用:
```c++
std::getline(std::istream& input, std::string& str, char delim);
```
如果你使用的是较老的编译器,或者你需要在不同的编译器之间进行兼容性测试,你可以使用这种形式的 `std::getline`。但是请注意,这种形式的 `std::getline` 在读取空行时可能会出现问题,因为它无法区分空行和行结束符。如果你需要处理空行,建议使用 C++11 标准或以上的版本。
相关问题
#include <iostream> #include <cstdio> #include <cstring> using namespace std; class STRING{ char* pstr; public: STRING(const char* s="");//已实现 ~STRING();//已实现 friend istream& operator>>(istream& in, STRING& s); friend ostream& operator<<(ostream& os, const STRING& s); }; int main() { STRING s; cin >> s; cout << s << endl; return 0; } /* 请在这里填写答案,,你只需要实现输入输出运算符(>>、<<)的重载即可 */
可以这样实现输入输出运算符的重载:
```c++
#include <iostream>
#include <cstdio>
#include <cstring>
using namespace std;
class STRING{
char* pstr;
public:
STRING(const char* s="");
~STRING();
friend istream& operator>>(istream& in, STRING& s);
friend ostream& operator<<(ostream& os, const STRING& s);
};
STRING::STRING(const char* s) {
pstr = new char[strlen(s)+1];
strcpy(pstr, s);
}
STRING::~STRING() {
delete []pstr;
}
istream& operator>>(istream& in, STRING& s) {
char buffer[1024];
in.getline(buffer, 1024);
s.pstr = new char[strlen(buffer)+1];
strcpy(s.pstr, buffer);
return in;
}
ostream& operator<<(ostream& os, const STRING& s) {
os << s.pstr;
return os;
}
int main() {
STRING s;
cin >> s;
cout << s << endl;
return 0;
}
```
在输入运算符中,我们使用`getline()`函数来读取输入的字符串,然后动态分配空间并将字符串拷贝到对象的数据成员中。
在输出运算符中,我们直接输出对象的数据成员。
注意,在使用动态分配空间时,需要在析构函数中释放空间。
“std::getline”: 未找到匹配的重载函数
如果你在编写程序时遇到了“std::getline: 未找到匹配的重载函数”的错误,可能是因为你的参数列表有误或者你的编译器不支持使用该函数。
请确保你的程序中包含以下代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
int main()
{
std::string line;
std::getline(std::cin, line); // 或者从文件中读取,如 std::getline(std::ifstream, line);
std::cout << "You entered: " << line << std::endl;
return 0;
}
```
如果你仍然遇到问题,可能是因为你的参数列表有误。请仔细检查输入参数的类型和数量是否符合函数定义。`std::getline` 的参数列表如下:
```c++
std::getline(std::basic_istream<CharT,Traits>& input, std::basic_string<CharT,Traits,Allocator>& str, CharT delim);
```
其中 `CharT` 为字符类型,`Traits` 为字符特性类型,`Allocator` 为分配器类型。如果你使用的是 `std::string` 类型,则 `CharT` 和 `Traits` 都为 `char`, `Allocator` 为 `std::allocator<char>`。
如果你使用的是较老的编译器,可能不支持该函数的某些特性。你可以尝试升级编译器或者使用其他的函数来读取输入流中的数据,例如 `std::cin >> line`。
阅读全文
相关推荐
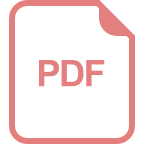
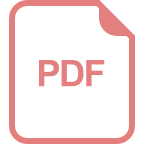


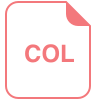
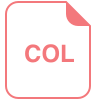
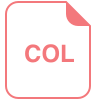
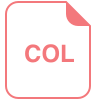
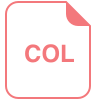







