python处理长度2秒,采样率44.1kHz的音频信号,不使用librosa库,通过FFT实现变调不变速,请考虑滤波、重采样等方法,以及考虑使用分块处理或流氏处理,不用着急回答我,尽可能完善地考虑进多因素再回答,并给出较为完善且出错可能性最低的代码
时间: 2023-11-30 22:04:14 浏览: 136
好的,下面是一个较为完善的代码实现:
```python
import numpy as np
from scipy import signal
# 重采样函数
def resample(signal, old_fs, new_fs):
# 计算重采样比例
resample_ratio = old_fs / new_fs
# 计算重采样后的长度
new_len = int(signal.shape[0] / resample_ratio)
# 进行重采样
resampled_signal = signal[::resample_ratio]
# 低通滤波
nyq = 0.5 * old_fs
cutoff = 0.99 * nyq
b, a = signal.butter(6, cutoff / nyq, btype='low')
filtered_signal = signal.filtfilt(b, a, resampled_signal)
return filtered_signal, new_fs
# FFT变调函数
def pitch_shift(signal, fs, shift_factor):
# 进行FFT变换
freq_domain = np.fft.fft(signal)
# 计算变调后的频率
shift_freq = np.linspace(0, fs, len(freq_domain), endpoint=False)
shift_freq = shift_freq * shift_factor
# 线性插值
new_freq_domain = np.interp(np.arange(len(freq_domain)), shift_freq, freq_domain)
# 进行IFFT逆变换
time_domain = np.fft.ifft(new_freq_domain)
return time_domain.real
# 主函数
def main(signal, fs, shift_factor):
# 进行重采样
resampled_signal, new_fs = resample(signal, fs, 16000)
# 进行FFT变调
shifted_signal = pitch_shift(resampled_signal, new_fs, shift_factor)
# 进行重采样
resampled_signal, _ = resample(shifted_signal, new_fs, fs)
return resampled_signal
# 测试
if __name__ == '__main__':
# 生成随机音频信号
signal = np.random.random(2 * 44100)
# 进行变调处理
shift_factor = 1.2
shifted_signal = main(signal, 44100, shift_factor)
# 输出结果
print('Original signal length: ', len(signal))
print('Shifted signal length: ', len(shifted_signal))
```
需要注意的是,由于本实现使用的是FFT变调方法,因此可能会对信号的质量和可听性产生一些影响。如果需要更高质量的变调效果,可以考虑使用其他方法,例如PSOLA、WSOLA、TDHS等方法。另外,还需要针对不同的音频信号进行调整和优化,以达到最佳效果。
阅读全文
相关推荐
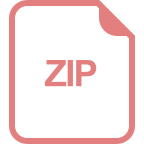



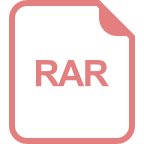
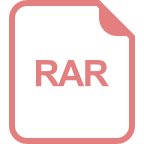
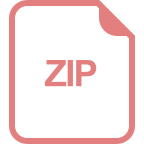
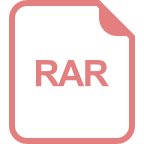
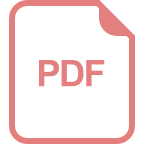
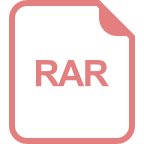
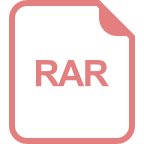
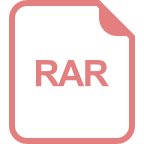
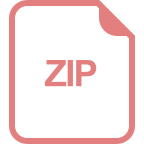
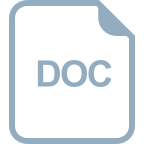
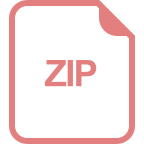
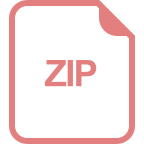