stm32蓝牙串口接收代码
时间: 2023-08-22 15:06:37 浏览: 45
好的,下面是一个简单的 STM32 蓝牙串口接收代码,你可以参考一下:
```c
#include "stm32f10x.h"
#include <stdio.h>
#define RX_BUF_SIZE 32
uint8_t rx_buf[RX_BUF_SIZE];
uint8_t rx_index = 0;
uint8_t rx_complete_flag = 0;
void USART1_IRQHandler(void)
{
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET) // 接收中断
{
uint8_t data = USART_ReceiveData(USART1); // 读取接收数据
if (data == '\n') // 如果接收到换行符,表示一条完整的数据已经接收完毕
{
rx_buf[rx_index] = '\0'; // 在接收缓冲区的末尾添加字符串结束符
rx_index = 0; // 重置接收缓冲区索引
rx_complete_flag = 1; // 标记接收完成
}
else
{
if (rx_index >= RX_BUF_SIZE) // 如果接收缓冲区已满,丢弃多余数据
{
rx_index = 0;
}
rx_buf[rx_index++] = data; // 将数据存入接收缓冲区
}
}
}
int main(void)
{
// 初始化 USART1
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
// 初始化 NVIC
NVIC_InitTypeDef NVIC_InitStructure;
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
while (1)
{
if (rx_complete_flag) // 如果接收完成
{
printf("Received data: %s\r\n", rx_buf); // 在串口终端打印接收到的字符串
rx_complete_flag = 0; // 清除接收完成标志位
}
}
}
```
这个代码通过 USART1 的 RX 中断来接收数据,当接收到换行符时,认为一条完整的数据已经接收完毕。如果接收缓冲区已满,会丢弃多余的数据,以确保接收缓冲区不会溢出。最终将接收到的字符串打印到串口终端上,以供调试和测试使用。
希望这个代码对你有所帮助!
相关推荐














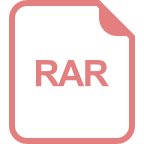