python如何替换csv第一列的数据
时间: 2024-05-01 18:18:51 浏览: 10
可以使用pandas库中的read_csv()函数将csv文件读入为一个DataFrame对象,然后将对应列的值替换成新的值,再使用to_csv()函数将DataFrame写入为新的csv文件。具体操作可以参考以下代码:
```python
import pandas as pd
# 读入csv文件为DataFrame对象
df = pd.read_csv('data.csv')
# 将第一列替换为新的值
df['col_name'] = df['col_name'].replace({'old_value': 'new_value'})
# 将修改后的DataFrame写入为新的csv文件
df.to_csv('new_data.csv', index=False)
```
其中,`col_name`为第一列的列名,`old_value`为需要替换的旧值,`new_value`为需要替换成的新值。最后的`index=False`表示不保存行索引。
相关问题
python替换csv中某行某列数据
你好!要使用 Python 替换 CSV 中某行某列的数据,你可以按照以下步骤进行:
1. 首先,导入 `csv` 模块,该模块提供了读写 CSV 文件的功能。
```python
import csv
```
2. 接下来,打开 CSV 文件并读取数据。假设你要替换的数据在第 3 行第 2 列,可以使用以下代码:
```python
with open('your_file.csv', 'r') as file:
reader = csv.reader(file)
rows = list(reader)
rows[2][1] = 'new_value'
```
这个代码片段将打开 CSV 文件,并使用 `csv.reader` 函数读取其中的数据。然后,将读取的数据存储在名为 `rows` 的列表中,并用索引 2 和 1 来访问第三行第二列的数据,并将其替换为新值。
3. 最后,将更改后的数据写回到 CSV 文件中。可以使用以下代码完成此操作:
```python
with open('your_file.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(rows)
```
这个代码片段将打开 CSV 文件,并使用 `csv.writer` 函数将更改后的数据写回到文件中。
完整的代码示例如下:
```python
import csv
with open('your_file.csv', 'r') as file:
reader = csv.reader(file)
rows = list(reader)
rows[2][1] = 'new_value'
with open('your_file.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(rows)
```
请记得将 `your_file.csv` 替换为你的 CSV 文件的实际文件名,并将索引值替换为你要替换的行和列的实际索引值。
python读取csv数据第一列
使用numpy中的read_csv函数来读取csv文件的数据,然后使用数组的方式来获取第一列的数据。具体代码如下所示:
```python
import numpy as np
# 读取csv文件
data = np.genfromtxt('stu_info.csv', delimiter=',', dtype=str)
# 获取第一列数据
first_column = data[:, 0]
# 打印第一列数据
print(first_column)
```
上述代码中,首先使用numpy中的genfromtxt函数读取csv文件的数据,然后使用数组的方式获取第一列数据,最后打印第一列数据。
相关推荐
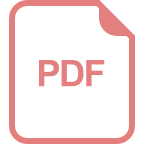
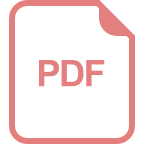












