在Adroid studio中写一段基于STMF103ZET6单片机通过蓝牙发送水温并接收实时显示的代码
时间: 2023-07-30 21:10:05 浏览: 214
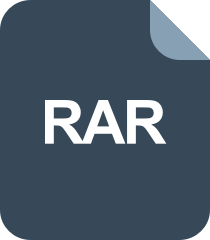
STM32F103ZET6步进电机智能小车蓝牙遥控+姿态角度显示程序源代码.rar

抱歉,Android Studio主要是用来开发Android应用程序的集成开发环境(IDE),不适合直接编写单片机程序。而且单片机程序需要使用C语言或汇编语言等低级语言进行编写,也需要使用单片机开发环境进行编译、下载和调试。
如果您想通过Android设备与单片机进行通信,可以使用Android设备上的蓝牙模块与单片机上的蓝牙模块进行通信。Android设备可以通过蓝牙串口协议(SPP)与单片机进行通信,发送和接收数据。以下是一个简单的Android应用程序的示例代码,可以通过蓝牙向单片机发送水温并显示实时数据:
```java
public class MainActivity extends AppCompatActivity {
private BluetoothAdapter mBluetoothAdapter;
private BluetoothSocket mBluetoothSocket;
private OutputStream mOutputStream;
private InputStream mInputStream;
private TextView mTextView;
private static final int REQUEST_ENABLE_BT = 1;
private static final UUID MY_UUID = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB");
private static final String DEVICE_ADDRESS = "00:11:22:33:44:55";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mTextView = (TextView) findViewById(R.id.textview);
mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
if (mBluetoothAdapter == null) {
Toast.makeText(this, "Device does not support Bluetooth", Toast.LENGTH_SHORT).show();
} else {
if (!mBluetoothAdapter.isEnabled()) {
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
} else {
connectToDevice();
}
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == REQUEST_ENABLE_BT) {
if (resultCode == RESULT_OK) {
connectToDevice();
} else {
Toast.makeText(this, "Bluetooth must be enabled to connect to device", Toast.LENGTH_SHORT).show();
}
}
}
private void connectToDevice() {
BluetoothDevice device = mBluetoothAdapter.getRemoteDevice(DEVICE_ADDRESS);
try {
mBluetoothSocket = device.createRfcommSocketToServiceRecord(MY_UUID);
mBluetoothSocket.connect();
mOutputStream = mBluetoothSocket.getOutputStream();
mInputStream = mBluetoothSocket.getInputStream();
startReadingData();
} catch (IOException e) {
e.printStackTrace();
}
}
private void startReadingData() {
new Thread(new Runnable() {
@Override
public void run() {
byte[] buffer = new byte[1024];
int bytes;
while (true) {
try {
bytes = mInputStream.read(buffer);
final String data = new String(buffer, 0, bytes);
runOnUiThread(new Runnable() {
@Override
public void run() {
mTextView.setText(data);
}
});
} catch (IOException e) {
e.printStackTrace();
break;
}
}
}
}).start();
}
public void sendTemperature(View view) {
// Replace with your own temperature reading code
int temperature = 25;
try {
mOutputStream.write(temperature);
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
protected void onDestroy() {
super.onDestroy();
try {
mBluetoothSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
需要注意,上述代码仅仅是一个示例,您需要根据自己的需求和单片机的实际情况进行修改和调试。同时,您还需要在单片机端编写程序来接收来自Android设备的数据,并进行相应的处理和显示。
阅读全文
相关推荐
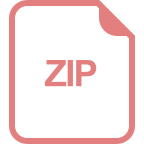
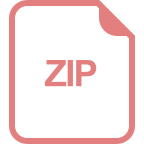






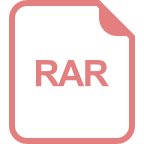
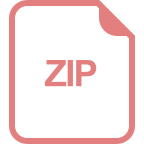





