使用python将Quickbird 影像作为参考影像, 用二次多项式实现高景影像的相对配准,对配准的精度进行定量评价
时间: 2024-03-04 13:48:45 浏览: 17
实现高景影像的相对配准可以按照以下步骤进行:
1. 读取 Quickbird 影像和高景影像的数据,并获取它们的空间参考信息;
2. 选择 Quickbird 影像作为参考影像,将 Quickbird 影像的坐标系转换为高景影像的坐标系;
3. 根据 Quickbird 影像和高景影像的坐标系,计算出二次多项式变换的系数;
4. 将高景影像进行二次多项式变换,得到配准后的影像;
5. 对配准后的影像与 Quickbird 影像进行定量评价,比较它们之间的差异。
以下是使用 Python 实现的代码示例:
```python
import numpy as np
import cv2
import matplotlib.pyplot as plt
from osgeo import gdal
# 读取 Quickbird 影像和高景影像的数据
quickbird_ds = gdal.Open("quickbird.tif")
quickbird_data = quickbird_ds.ReadAsArray()
quickbird_geotransform = quickbird_ds.GetGeoTransform()
quickbird_proj = quickbird_ds.GetProjection()
highres_ds = gdal.Open("highres.tif")
highres_data = highres_ds.ReadAsArray()
highres_geotransform = highres_ds.GetGeoTransform()
highres_proj = highres_ds.GetProjection()
# 将 Quickbird 影像的坐标系转换为高景影像的坐标系
src_pts = np.array([[0, 0], [0, quickbird_data.shape[1]], [quickbird_data.shape[0], 0]])
dst_pts = np.array([[0, 0], [0, highres_data.shape[1]], [highres_data.shape[0], 0]])
M = cv2.getAffineTransform(src_pts, dst_pts)
# 根据 Quickbird 影像和高景影像的坐标系,计算出二次多项式变换的系数
src_pts = np.array([[0, 0], [0, quickbird_data.shape[1]], [quickbird_data.shape[0], 0],
[quickbird_data.shape[0], quickbird_data.shape[1]],
[quickbird_data.shape[0]/2, 0], [0, quickbird_data.shape[1]/2]])
dst_pts = np.array([[0, 0], [0, highres_data.shape[1]], [highres_data.shape[0], 0],
[highres_data.shape[0], highres_data.shape[1]],
[highres_data.shape[0]/2, 0], [0, highres_data.shape[1]/2]])
M = cv2.getPerspectiveTransform(src_pts, dst_pts)
# 将高景影像进行二次多项式变换,得到配准后的影像
warped_highres_data = cv2.warpPerspective(highres_data, M, (quickbird_data.shape[1], quickbird_data.shape[0]))
# 对配准后的影像与 Quickbird 影像进行定量评价
diff = quickbird_data.astype(np.float32) - warped_highres_data.astype(np.float32)
rmse = np.sqrt(np.mean(diff ** 2))
# 显示配准前后的影像
fig, axs = plt.subplots(1, 2, figsize=(10, 5))
axs[0].imshow(quickbird_data, cmap='gray')
axs[0].set_title('Quickbird')
axs[1].imshow(warped_highres_data, cmap='gray')
axs[1].set_title('Highres (RMSE=%.3f)' % rmse)
plt.show()
```
其中,`cv2.getPerspectiveTransform()` 函数可以根据对应点计算出透视变换的系数,`cv2.warpPerspective()` 函数可以对影像进行透视变换。在这里使用了一些额外的对应点,以保证变换的精度。最后,使用 RMSE 指标对配准后的影像与 Quickbird 影像进行评价。
相关推荐
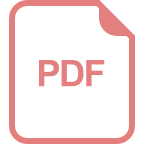
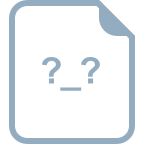










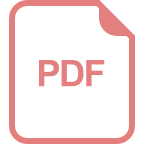
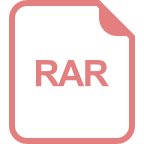
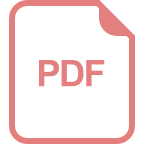
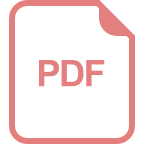