根据错误:AttributeError: module 'networkx' has no attribute 'from_numpy_matrix',修改下述代码:import os import jieba.analyse from textrank4zh import TextRank4Keyword import concurrent.futures # 定义分块读取函数 def read_in_chunks(file_path, chunk_size=1024*1024): with open(file_path, 'r', encoding='utf-8') as f: while True: data = f.read(chunk_size) if not data: break yield data # 定义处理函数 def process_chunk(chunk): # 使用jieba分词提取关键词 jieba_keywords = jieba.analyse.extract_tags(chunk, topK=10, withWeight=True) # 使用textrank4zh提取关键词 tr4w = TextRank4Keyword() tr4w.analyze(chunk, lower=True, window=2) textrank_keywords = tr4w.get_keywords(10, word_min_len=2) # 合并两种方法提取的关键词 keywords = jieba_keywords + textrank_keywords return keywords # 读取文本文件,并按块处理 chunks = [] for chunk in read_in_chunks('input.txt'): chunks.append(chunk) # 多线程并行处理 results = [] with concurrent.futures.ThreadPoolExecutor() as executor: futures = [executor.submit(process_chunk, chunk) for chunk in chunks] for future in concurrent.futures.as_completed(futures): results.extend(future.result()) # 合并结果,并按权重降序排序 keywords = {} for keyword, weight in results: if keyword in keywords: keywords[keyword] += weight else: keywords[keyword] = weight keywords = sorted(keywords.items(), key=lambda x: x[1], reverse=True) keywords = [(keyword, weight) for keyword, weight in keywords if len(keyword) > 1][:10] # 输出到txt文件中 with open('output.txt', 'w', encoding='utf-8') as f: for keyword, weight in keywords: f.write(keyword + '\t' + str(weight) + '\n')
时间: 2024-03-03 10:50:56 浏览: 208
根据错误信息 `AttributeError: module 'networkx' has no attribute 'from_numpy_matrix'`,可能是因为缺少 `networkx` 模块的相关依赖库。可以尝试安装一下这些依赖库,例如 `scipy` 库。
修改后的代码如下:
```python
import os
import jieba.analyse
from textrank4zh import TextRank4Keyword
import concurrent.futures
import scipy
# 定义分块读取函数
def read_in_chunks(file_path, chunk_size=1024*1024):
with open(file_path, 'r', encoding='utf-8') as f:
while True:
data = f.read(chunk_size)
if not data:
break
yield data
# 定义处理函数
def process_chunk(chunk):
# 使用jieba分词提取关键词
jieba_keywords = jieba.analyse.extract_tags(chunk, topK=10, withWeight=True)
# 使用textrank4zh提取关键词
tr4w = TextRank4Keyword()
tr4w.analyze(chunk, lower=True, window=2)
textrank_keywords = tr4w.get_keywords(10, word_min_len=2)
# 合并两种方法提取的关键词
keywords = jieba_keywords + textrank_keywords
return keywords
# 读取文本文件,并按块处理
chunks = []
for chunk in read_in_chunks('input.txt'):
chunks.append(chunk)
# 多线程并行处理
results = []
with concurrent.futures.ThreadPoolExecutor() as executor:
futures = [executor.submit(process_chunk, chunk) for chunk in chunks]
for future in concurrent.futures.as_completed(futures):
results.extend(future.result())
# 合并结果,并按权重降序排序
keywords = {}
for keyword, weight in results:
if keyword in keywords:
keywords[keyword] += weight
else:
keywords[keyword] = weight
keywords = sorted(keywords.items(), key=lambda x: x[1], reverse=True)
keywords = [(keyword, weight) for keyword, weight in keywords if len(keyword) > 1][:10]
# 输出到txt文件中
with open('output.txt', 'w', encoding='utf-8') as f:
for keyword, weight in keywords:
f.write(keyword + '\t' + str(weight) + '\n')
```
请注意,如果还存在其他错误,需要根据具体的错误信息进行相应的修改。
阅读全文
相关推荐
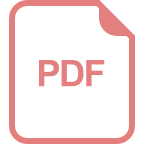
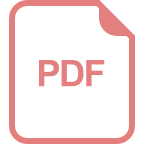
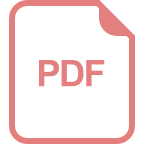















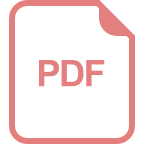