C语言根据元素关键字的值删除元素
时间: 2024-06-11 11:04:55 浏览: 49
以下是一个示例程序,可以根据元素关键字的值删除元素:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 10
struct element {
int key;
char name[10];
};
struct element array[MAX_SIZE];
int count = 0;
void add_element(int key, char *name) {
if (count < MAX_SIZE) {
array[count].key = key;
strcpy(array[count].name, name);
count++;
} else {
printf("Array is full!\n");
}
}
void remove_element(int key) {
int i, j;
for (i = 0; i < count; i++) {
if (array[i].key == key) {
for (j = i; j < count - 1; j++) {
array[j] = array[j+1];
}
count--;
return;
}
}
printf("Element with key %d not found!\n", key);
}
void print_array() {
int i;
printf("Array contents:\n");
for (i = 0; i < count; i++) {
printf(" Key: %d, Name: %s\n", array[i].key, array[i].name);
}
}
int main() {
add_element(1, "Alice");
add_element(2, "Bob");
add_element(3, "Charlie");
add_element(4, "Dave");
print_array();
remove_element(2);
print_array();
remove_element(5);
return 0;
}
```
该程序定义了一个结构体元素,包含一个关键字和一个名称。使用一个全局数组来存储元素,同时记录元素的数量count。程序提供了三个函数:add_element用于添加元素,remove_element用于删除元素,print_array用于打印数组内容。remove_element函数遍历数组,查找关键字等于给定值的元素,然后将该元素之后的元素向前移动一个位置,最后将count减1。如果找不到给定关键字的元素,函数将打印一条错误消息。
在main函数中,我们添加了四个元素,然后打印数组,删除关键字为2的元素,再次打印数组,最后尝试删除关键字为5的元素(该元素不存在)。运行程序将输出以下内容:
```
Array contents:
Key: 1, Name: Alice
Key: 2, Name: Bob
Key: 3, Name: Charlie
Key: 4, Name: Dave
Array contents:
Key: 1, Name: Alice
Key: 3, Name: Charlie
Key: 4, Name: Dave
Element with key 5 not found!
```
阅读全文
相关推荐













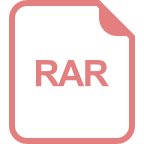




