clahe算法python实现
时间: 2023-07-26 18:33:44 浏览: 134
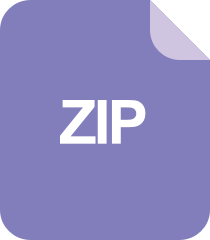
基于Python算法实现
在Python中,可以使用OpenCV库来实现CLAHE算法。具体步骤如下:
1. 读入图像并将其转换为灰度图像。
```python
import cv2
# 读入图像
img = cv2.imread('input.jpg')
# 将图像转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. 创建CLAHE对象并设置参数。
```python
# 创建CLAHE对象并设置参数
clahe = cv2.createCLAHE(clipLimit=2.0, tileGridSize=(8,8))
```
其中,`clipLimit`表示对比度增强的限制因子,`tileGridSize`表示将图像分割为多少个小块进行处理。
3. 对图像进行直方图均衡化。
```python
# 对灰度图像进行直方图均衡化
gray_clahe = clahe.apply(gray)
```
4. 显示结果。
```python
# 显示结果
cv2.imshow('original', img)
cv2.imshow('CLAHE', gray_clahe)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
完整代码如下:
```python
import cv2
# 读入图像
img = cv2.imread('input.jpg')
# 将图像转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 创建CLAHE对象并设置参数
clahe = cv2.createCLAHE(clipLimit=2.0, tileGridSize=(8,8))
# 对灰度图像进行直方图均衡化
gray_clahe = clahe.apply(gray)
# 显示结果
cv2.imshow('original', img)
cv2.imshow('CLAHE', gray_clahe)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
注意,在显示图像时,使用`cv2.imshow()`函数。`cv2.waitKey(0)`用于等待用户按下键盘,`cv2.destroyAllWindows()`用于关闭所有窗口。
阅读全文
相关推荐
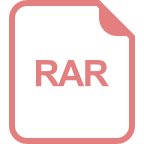
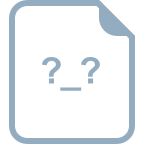

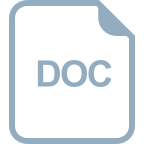
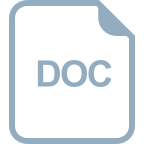










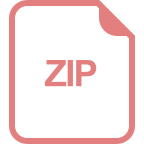

