pytorch grad-cam代码
时间: 2023-05-31 21:19:55 浏览: 217
### 回答1:
我不太确定这个问题的具体内容,但是我可以简单介绍一下PyTorch Grad-CAM代表的含义和相关代码。Grad-CAM是一种可视化技术,可以帮助我们理解模型的决策过程。在PyTorch中,Grad-CAM代码可以通过对预测结果进行反向传播,然后根据传播的梯度信息计算特定部位的重要性,从而得出图像各部位对于预测结果的贡献程度。相关代码可以通过PyTorch库中的torch.nn.functional.interpolate函数和cv2库中的resize函数来实现。
### 回答2:
Grad-CAM是一种可视化卷积神经网络中的重要区域的方法,可以评估模型学习(分类)的可解释性。PyTorch是一种用于构建深度学习模型的开源框架,支持灵活的计算图形构建、自动求导和动态图形优化。在这篇回答中,我们将讨论如何使用PyTorch实现Grad-CAM。
首先需要安装必要的Python包,如PyTorch、NumPy和PIL。接下来,我们需要构建一个PyTorch模型,用于进行分类任务。再次提醒,模型需要以计算图的形式定义。
现在,我们需要实现Grad-CAM。Grad-CAM的思想是在给定输入图像和类别后,计算出特定类别对每个特征图的重要性分数。这可以通过计算由类别分数得到的梯度并在特征图上评估梯度的平均值来实现。以下是Grad-CAM的代码:
``` python
def grad_cam(model, input, class_idx, layer_name):
# get the features based on the input tensor
features = model.features(input)
# get the output of the classifier based on the features
output = model.classifier(features)
# zero the gradients
model.zero_grad()
# compute the gradient of the output category with respect to feature map
output[:, class_idx].backward(retain_graph=True)
# get the feature activations
activations = model.features[layer_name].forward(input)
# compute the importance map
importance_map = torch.mean(torch.tensor(activations.grad[0]), axis=(1, 2)).detach().numpy()
# apply RELU to the importance map
importance_map = np.maximum(importance_map, 0)
# resize the importance map to the input shape
importance_map = cv2.resize(importance_map, input.shape[2:])
# normalize the importance map
importance_map = (importance_map - np.min(importance_map)) / (np.max(importance_map) - np.min(importance_map))
return importance_map
```
在代码中,我们首先提取给定输入的特征。接下来,我们计算由给定类别得到的梯度,并根据这些梯度计算特征图的重要性分数。然后,我们使用ReLU激活并调整重要性分数的大小,使其与给定输入匹配。最后,我们返回标准化的重要性映射。
执行Grad-CAM后,我们需要将结果显示在输入图像上。以下是一个简单的例子:
``` python
input, label = dataset[0]
class_idx = label.item()
layer_name = 'conv5/relu'
importance_map = grad_cam(model, input, class_idx, layer_name)
img = input.numpy().transpose((1, 2, 0))
plt.imshow(img)
plt.imshow(importance_map, alpha=0.5, cmap='jet')
```
在代码段中,我们首先获取输入张量和目标类别。然后,我们指定一个带ReLU的层(即最后一个卷积层),并使用Grad-CAM计算重要性映射。最后,我们将输入张量可视化,并将重要性映射叠加在上面。
在这个例子中,我们使用一个简单的CNN进行图像分类。使用类似的方法,我们可以对任何模型和任何图像进行Grad-CAM计算。因此,通过使用PyTorch,我们可以方便地实现和理解Grad-CAM。
### 回答3:
PyTorch Grad-CAM是一种可视化技术,通过将卷积神经网络的特征图与最终输出相结合,可以确定预测的重要区域。Grad-CAM代表梯度加权类激活图,它利用梯度信息将网络层的重要性映射到输入图像上,使得可以直观地理解卷积神经网络的决策。该技术使得我们可以以像素级别确定模型重点关注区域,以用于调试、可视化和解释该模型如何进行分类决策。
下面是一个使用PyTorch实现Grad-CAM的代码:
```
import torch
import torch.nn as nn
from torch.autograd import Variable
from torchvision import models, transforms
import cv2
import numpy as np
import sys
class CamExtractor():
""" Class for extracting activations and
registering gradients from targetted intermediate layers """
def __init__(self, model, target_layers):
self.model = model
self.target_layers = target_layers
self.gradients = []
def save_gradient(self, grad):
self.gradients.append(grad)
def forward_pass(self, x):
""" Does a forward pass on convolutions, hooks the
activations and gradients """
conv_output = None
for name, module in self.model.named_modules():
x = module(x)
if name in self.target_layers:
x.register_hook(self.save_gradient)
conv_output = x
return conv_output, x
class GradCam():
def __init__(self, model, target_layers, use_cuda):
self.model = model
self.model.eval()
self.cuda = use_cuda
if self.cuda:
self.model = model.cuda()
self.extractor = CamExtractor(self.model, target_layers)
def forward(self, input):
return self.model(input)
def __call__(self, input, index=None):
"""Generates class activation map for the input image"""
if self.cuda:
features, output = self.extractor.forward_pass(input.cuda())
else:
features, output = self.extractor.forward_pass(input)
if index == None:
index = np.argmax(output.cpu().data.numpy())
one_hot = np.zeros((output.size()[-1]), dtype=np.float32)
one_hot[index] = 1
one_hot = Variable(torch.from_numpy(one_hot), requires_grad=True)
if self.cuda:
one_hot = one_hot.cuda()
one_hot = torch.sum(one_hot * output)
self.model.zero_grad()
one_hot.backward()
self.weights = self.extractor.gradients[-1].mean(dim=(-1, -2), keepdim=True)
cam = torch.sum(self.weights * features, dim=1).squeeze()
cam_relu = np.maximum(cam.cpu().data.numpy(), 0)
cam_relu = cam_relu / np.max(cam_relu)
return cam_relu
if __name__ == '__main__':
# define the model
model = models.resnet50(pretrained=True)
grad_cam = GradCam(model=model, target_layers=['layer4'], use_cuda=True)
# load and preprocess an input image
img = cv2.imread('input.jpg')
img = cv2.resize(img, (224, 224))
img = np.float32(img) / 255
input = transforms.ToTensor()(img).unsqueeze(0)
# use the grad cam class to generate the heat map
cam = grad_cam(input)
# use OpenCV to apply the heat map to the input image
heatmap = cv2.applyColorMap(np.uint8(255 * cam), cv2.COLORMAP_JET)
heatmap = np.float32(heatmap) / 255
cam = heatmap + np.float32(img)
cam = cam / np.max(cam)
# save the output
cv2.imwrite("cam.jpg", np.uint8(255 * cam))
```
在该代码中,我们使用了PyTorch的模型和变换,其中包括了ResNet-50模型。我们在GradCam类中定义了一个前向函数,将输入图片传递给该函数,该函数返回模型输出。然后我们通过计算模型中所有运行层的输出特征,直到我们找到了我们感兴趣的“target layer”,并将它们注册到我们的“CamExtractor”类中。接下来,我们定义了一个“__call__”函数来执行Grad-CAM算法。它首先执行前向传递和后向传递,并计算权重。权重是特征图的梯度取平均值。接下来,我们将权重分别乘以特征图并在通道维进行求和,这返回一个二维的热力图。最后,我们使用OpenCV应用热图进行可视化。
相关推荐
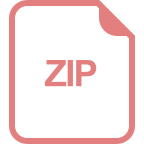














