pageresult pageresult = new pageresult(goodslist, total, pageutil.getlimit()
时间: 2023-05-13 21:01:27 浏览: 55
这段代码实例化了一个名为pageresult的对象,它的构造函数需要传入三个参数:goodslist、total和pageutil.getlimit()。其中goodslist表示商品列表,total表示商品总数,pageutil.getlimit()表示每页显示的商品数量。这个对象的作用是将商品列表根据分页规则进行分页,并返回当前页面所需的商品数据。在进行分页操作时,需要提供当前页码、每页显示的商品数量等参数,这些参数可以通过调用pageresult对象的不同方法来获取。使用这个对象可以方便地实现商品列表的分页展示。
相关问题
// 商品排序 sortPrice() { if (this.value == 0) { this.goodsList = this.goodsList.sort((a, b) => { // console.log("000");0 return a.id - b.id; }); } else if (this.value == 1) { this.goodsList = this.goodsList.sort((a, b) => { // console.log(111); return b.price - a.price; }); } else if (this.value == 2) { this.goodsList = this.goodsList.sort((a, b) => { // console.log(222); return a.price - b.price; }); } else if (this.value == 3) { this.goodsList = this.goodsList.sort((a, b) => { // console.log(333); return b.volume - a.volume; }); } else if (this.value == 4) { this.goodsList = this.goodsList.sort((a, b) => { // console.log(444); return a.volume - b.volume; }); } }, // 点击有货优先时的回调函数 isHaveGoods() { // console.log(5555); // 只保留有货的商品 this.goodsList = this.goodsList.sort((a, b) => { return b.isHave - a.isHave; }); }, // 直营优先 isDirectGoods() { // console.log(6666); // 只保留有货的商品 this.goodsList = this.goodsList.sort((a, b) => { return b.isDirect - a.isDirect; }); },怎么把价格排序和直营优先排序结合起来
要把价格排序和直营优先排序结合起来,可以按照以下步骤进行:
1. 在前端页面上增加排序按钮或下拉菜单,包括价格排序和直营优先排序的选项。
2. 当用户选择价格排序选项时,调用 `sortPrice()` 函数进行价格排序。当用户选择直营优先排序选项时,调用 `isDirectGoods()` 函数进行直营优先排序。
3. 在 `sortPrice()` 函数中,增加直营优先的筛选条件,使排序结果中直营商品排在前面。具体实现可以在排序前先筛选出直营商品,然后再对直营商品和非直营商品分别进行价格排序,最后把排序后的直营商品和非直营商品合并起来。
4. 在 `isDirectGoods()` 函数中,增加价格排序的筛选条件,使排序结果中直营商品和非直营商品均按价格排序。具体实现可以先筛选出直营商品和非直营商品,然后分别对直营商品和非直营商品进行价格排序,最后把排序后的直营商品和非直营商品合并起来。
例如,在 `sortPrice()` 函数中可以按以下方式实现价格排序和直营优先排序的结合:
```javascript
sortPrice() {
if (this.value == 0) {
// 按商品ID排序
this.goodsList = this.goodsList.sort((a, b) => {
return a.id - b.id;
});
} else if (this.value == 1) {
// 按价格从高到低排序
let directGoods = this.goodsList.filter(item => item.isDirect);
let notDirectGoods = this.goodsList.filter(item => !item.isDirect);
directGoods.sort((a, b) => b.price - a.price);
notDirectGoods.sort((a, b) => b.price - a.price);
this.goodsList = directGoods.concat(notDirectGoods);
} else if (this.value == 2) {
// 按价格从低到高排序
let directGoods = this.goodsList.filter(item => item.isDirect);
let notDirectGoods = this.goodsList.filter(item => !item.isDirect);
directGoods.sort((a, b) => a.price - b.price);
notDirectGoods.sort((a, b) => a.price - b.price);
this.goodsList = directGoods.concat(notDirectGoods);
} else if (this.value == 3) {
// 按销量从高到低排序
this.goodsList = this.goodsList.sort((a, b) => {
return b.volume - a.volume;
});
} else if (this.value == 4) {
// 按销量从低到高排序
this.goodsList = this.goodsList.sort((a, b) => {
return a.volume - b.volume;
});
}
},
```
在 `isDirectGoods()` 函数中可以按以下方式实现价格排序和直营优先排序的结合:
```javascript
isDirectGoods() {
if (this.value == 1) {
// 直营优先,按价格从高到低排序
let directGoods = this.goodsList.filter(item => item.isDirect);
let notDirectGoods = this.goodsList.filter(item => !item.isDirect);
directGoods.sort((a, b) => b.price - a.price);
notDirectGoods.sort((a, b) => b.price - a.price);
this.goodsList = directGoods.concat(notDirectGoods);
} else if (this.value == 2) {
// 直营优先,按价格从低到高排序
let directGoods = this.goodsList.filter(item => item.isDirect);
let notDirectGoods = this.goodsList.filter(item => !item.isDirect);
directGoods.sort((a, b) => a.price - b.price);
notDirectGoods.sort((a, b) => a.price - b.price);
this.goodsList = directGoods.concat(notDirectGoods);
} else {
// 直营优先,按直营优先排序
this.goodsList = this.goodsList.sort((a, b) => {
return b.isDirect - a.isDirect;
});
}
}
```
这样,就可以实现在点击其他筛选条件的时候还能进行价格由高到低或由低到高的排序,同时也能够实现直营优先的排序。
代码解释 computed: { // 小选全选 checkedall: { set(value) { this.goodsList.forEach((item) => { return (item.checked = value); }); }, get() { return this.goodsList.every((item) => { return item.checked == true; }); }, }, // 合计 total: { set() {}, get() { let num = 0; this.goodsList.forEach((item) => { if (item.checked == true) { num += item.price * item.num; } }); return num; }, }, //数量 number: { set() {}, get() { let allnum = 0; this.goodsList.forEach((item) => { if (item.checked == true) { allnum += item.num; } }); return allnum; }, }, },
这段代码是Vue中的computed计算属性,其中包含三个计算属性:
1. `checkedall`:用于实现全选/全不选的功能。当我们点击全选按钮时,会将`checkedall`的值改为true,同时将商品列表中的每一项的`checked`属性都设置为true;当我们点击全不选按钮时,会将`checkedall`的值改为false,同时将商品列表中的每一项的`checked`属性都设置为false。该计算属性的`get`方法用于判断是否所有的商品都被选中。
2. `total`:用于计算所有选中商品的总价。当商品的`checked`属性为true时,会将该商品的价格乘以数量累加到总价中。该计算属性的`get`方法返回总价。
3. `number`:用于计算所有选中商品的数量。当商品的`checked`属性为true时,会将该商品的数量累加到总数量中。该计算属性的`get`方法返回总数量。
这三个计算属性都是只读属性,不能直接修改,需要通过修改商品列表中每一项的`checked`属性来触发计算属性的更新。
相关推荐
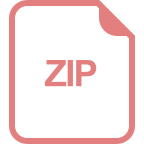











<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@page import="com.test.bean.Goods,java.util.ArrayList" %> <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>购物车</title> <style type="text/css"> table{border-collapse:collapse;} td{border:1px solid black; text-align:center; } #deal{margin-left:200px} </style> </head> <body> <jsp:useBean id="cart" class="com.test.bean.Cart" scope="session"></jsp:useBean> <%if(cart==null||cart.getGoodslist().size()==0) out.println("购物车空空如也.....返回商品首页"); else{ ArrayList<Goods>goodslist=cart.getGoodslist(); %> 当前购物车共有<%=cart.getGcount() %>件物品 返回商品首页
序号 商品号 商品名称 价格 数量 小计 描述 <%for(int i=0;i<goodslist.size();i++){ Goods goods=goodslist.get(i); %> <%=i+1 %> <%=goods.getGid() %> <%=goods.getGname() %> <%= String.format("%.2f", goods.getGprice())%> <%=goods.getGcount() %> <%=String.format("%.2f", goods.getGprice()*goods.getGcount()) %> 删除 <%}%>
总计<%=String.format("%.2f",cart.getTotal())%> <input type="button" id="deal" value="购买" /></input> <%}%> <script type="text/javascript"> var dealbutton=document.getElementById("deal"); dealbutton.onclick=function(){ var cf=confirm("确定购买吗?"); if(cf==true){ window.location.href="Servlet/DealServlet"; } } </script> </body> </html> 修改删除功能,在购物车物品数量大于1的情况下点击“删除”时减少1个,等于1时删除此项商品;

