c++编程,定义能计算的日期类,当日期递增到新的一天时,能够修改日期。(提示需要考虑闰年,年末,月末的情况,)测试数据中默认基准日期为2000年1月1日,当前日期为输入数据,365天是输入天数,输出当前日期过后365天的日期。毕业日期为输入日期,需计算与当前日期之间相隔天数。
时间: 2023-12-07 14:03:28 浏览: 106
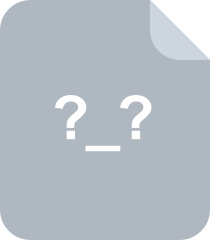
C#计算两个日期间隔的年月日,精确到天,无视闰年等
好的,这是一个关于日期类的编程问题。我可以给出一个基本的实现方案,你可以根据实际情况进行修改和补充。
首先,定义一个日期类,包含年、月、日三个成员变量,以及一些方法和运算符重载,用于日期的计算和比较。
```c++
class Date {
public:
Date(int year = 2000, int month = 1, int day = 1);
bool operator<(const Date& other) const;
bool operator==(const Date& other) const;
Date operator+(int days) const;
int operator-(const Date& other) const;
void increment();
void print() const;
private:
int year_;
int month_;
int day_;
static const int days_per_month_[13];
};
```
其中,构造函数用于初始化日期,运算符重载用于日期的比较和计算,increment() 方法用于将日期递增一天,print() 方法用于输出日期的字符串表示。
为了实现日期的递增,我们需要考虑以下几种情况:
1. 当前日期是年末,需要将年份加 1,月份和日份归零。
2. 当前日期是月末,需要将月份加 1,日份归零。
3. 当前日期是闰年 2 月 29 日,需要特殊处理。
具体实现可以参考以下代码:
```c++
const int Date::days_per_month_[13] = {0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
Date::Date(int year, int month, int day) {
year_ = year;
month_ = month;
day_ = day;
}
bool Date::operator<(const Date& other) const {
if (year_ != other.year_) {
return year_ < other.year_;
} else if (month_ != other.month_) {
return month_ < other.month_;
} else {
return day_ < other.day_;
}
}
bool Date::operator==(const Date& other) const {
return year_ == other.year_ && month_ == other.month_ && day_ == other.day_;
}
Date Date::operator+(int days) const {
Date result = *this;
for (int i = 0; i < days; i++) {
result.increment();
}
return result;
}
int Date::operator-(const Date& other) const {
int days = 0;
Date date = *this;
while (date < other) {
days--;
date.increment();
}
while (other < date) {
days++;
other.increment();
}
return days;
}
void Date::increment() {
if (day_ == days_per_month_[month_]) { // end of month
if (month_ == 12) { // end of year
year_++;
month_ = 1;
day_ = 1;
} else {
month_++;
day_ = 1;
}
} else if (month_ == 2 && day_ == 28 && (year_ % 4 == 0 && year_ % 100 != 0 || year_ % 400 == 0)) { // leap year
day_++;
} else {
day_++;
}
}
void Date::print() const {
printf("%04d-%02d-%02d", year_, month_, day_);
}
```
接下来,我们可以编写主函数,读入输入数据,计算输出结果:
```c++
int main() {
int year, month, day, days;
scanf("%d-%d-%d %d", &year, &month, &day, &days);
Date start_date(year, month, day);
Date end_date = start_date + days;
end_date.print();
printf("\n");
Date graduation_date(2022, 6, 30);
int days_left = graduation_date - start_date;
printf("%d\n", days_left);
return 0;
}
```
这样,我们就完成了这个问题的基本实现。你可以根据需要进行修改和扩展。
阅读全文
相关推荐
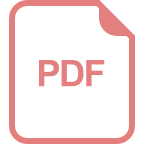
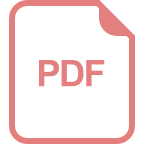















