1. 编写程序(多重继承):定义一个动物类Animal,成员变量包括:int型的m_nWeight(重量)。成员函数包括:SetWeight(int nWeight)、GetWeight() 。由Animal类派生出马类Horse,添加成员函数:Run() { cout<<"I can run"<<endl; } 由Animal类派生出鸟类Bird,添加成员函数:Fly() { cout<<"I can fly"<<endl; } ,由Horse类和Bird类共同派生出天马类Pegasus。 要求:主函数中创建一个Pegasus类对象,执行run和fly操作,并设置体重输出。
时间: 2024-03-22 13:39:08 浏览: 98
以下是一种实现方式:
```c++
#include <iostream>
using namespace std;
class Animal {
protected:
int m_nWeight;
public:
void SetWeight(int nWeight) {
m_nWeight = nWeight;
}
int GetWeight() {
return m_nWeight;
}
};
class Horse : public Animal {
public:
void Run() {
cout << "I can run" << endl;
}
};
class Bird : public Animal {
public:
void Fly() {
cout << "I can fly" << endl;
}
};
class Pegasus : public Horse, public Bird {
public:
void RunAndFly() {
Horse::Run();
Bird::Fly();
}
};
int main() {
Pegasus pegasus;
pegasus.SetWeight(100);
pegasus.RunAndFly();
cout << "My weight is " << pegasus.GetWeight() << endl;
return 0;
}
```
在这个程序中,定义了 Animal 类作为基类,Horse 类和 Bird 类分别从 Animal 类派生出来,Pegasus 类从 Horse 和 Bird 类共同派生出来。Pegasus 类中定义了 RunAndFly() 成员函数,该函数调用 Horse 类和 Bird 类中的函数来实现 Pegasus 类的功能。在主函数中创建一个 Pegasus 类对象,设置其体重并调用 RunAndFly() 函数来执行 run 和 fly 操作。最后输出 Pegasus 类对象的体重。
阅读全文
相关推荐
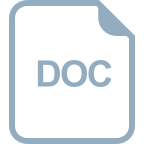
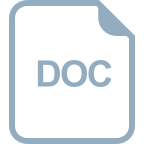
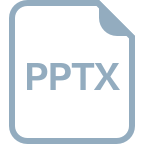







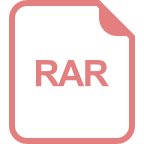
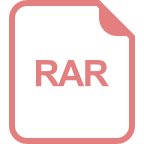
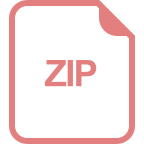
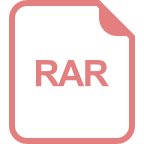
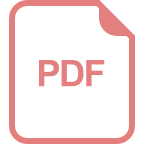
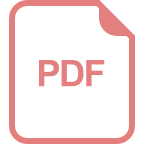
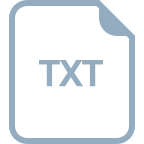