Android如何将html转换为json代码
时间: 2024-02-12 15:03:56 浏览: 36
在Android中将HTML转换为JSON需要使用第三方的库来实现。以下是一个示例代码,演示如何使用jsoup库来解析HTML并将其转换为JSON格式:
```java
// 引入jsoup库
implementation 'org.jsoup:jsoup:1.14.2'
String htmlStr = "<html><head><title>Test</title></head><body><div><p>Hello World!</p></div></body></html>";
try {
// 将HTML字符串解析为Document对象
Document doc = Jsoup.parse(htmlStr);
// 遍历Document对象中的所有元素,并转换为JSONObject对象
JSONObject json = new JSONObject();
Element root = doc.child(0);
JSONObject jsonRoot = new JSONObject();
json.put(root.tagName(), jsonRoot);
for (Element e : root.getAllElements()) {
if (!e.tagName().equals(root.tagName())) {
JSONObject jsonElem = new JSONObject();
jsonElem.put("text", e.text());
for (Attribute attr : e.attributes()) {
jsonElem.put(attr.getKey(), attr.getValue());
}
if (jsonRoot.has(e.tagName())) {
JSONArray jsonArr = jsonRoot.getJSONArray(e.tagName());
jsonArr.put(jsonElem);
} else {
JSONArray jsonArr = new JSONArray();
jsonArr.put(jsonElem);
jsonRoot.put(e.tagName(), jsonArr);
}
}
}
// 打印转换后的JSON对象
Log.d("JSON", json.toString());
} catch (JSONException e) {
e.printStackTrace();
}
```
在上述示例代码中,首先将HTML字符串解析为一个Document对象,然后遍历Document对象中的所有元素,并将每个元素转换为一个JSONObject对象。具体来说,通过getAllElements方法获取所有元素,然后将元素的标签名作为JSONObject对象的键,将元素的文本和属性作为JSONObject对象的值。如果有多个同名的元素,将它们放在一个JSONArray对象中。最后将所有JSONObject对象放在一个大的JSONObject对象中,并打印出来。需要注意的是,由于HTML的结构和元素类型比较复杂,转换过程中需要根据具体情况进行一些特殊处理。
相关推荐
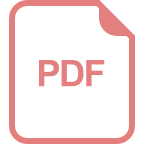
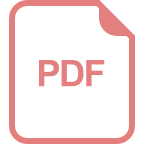
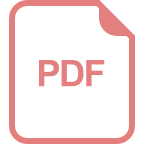














