Java实现文件按照60MB分卷压缩的单元测试
时间: 2023-03-02 09:16:06 浏览: 100
以下是一个Java实现文件按照60MB分卷压缩的单元测试的示例代码:
```java
import org.junit.Test;
import java.io.*;
public class FileCompressionTest {
@Test
public void testFileCompression() throws IOException {
String filePath = "path/to/your/file";
String outputDir = "path/to/your/output/dir";
// 创建输入文件和输出目录
File inputFile = new File(filePath);
File outputDirFile = new File(outputDir);
if (!inputFile.exists()) {
throw new FileNotFoundException("输入文件不存在");
}
if (!outputDirFile.exists()) {
outputDirFile.mkdirs();
}
// 读取输入文件并分卷压缩
int bufferSize = 1024;
int maxVolumeSize = 60 * 1024 * 1024; // 60MB
byte[] buffer = new byte[bufferSize];
int count;
int volumeIndex = 1;
try (FileInputStream fileInputStream = new FileInputStream(inputFile);
BufferedInputStream bufferedInputStream = new BufferedInputStream(fileInputStream)) {
while ((count = bufferedInputStream.read(buffer)) != -1) {
// 创建输出文件
String outputFilePath = outputDir + File.separator + inputFile.getName() + ".zip." + volumeIndex;
FileOutputStream fileOutputStream = new FileOutputStream(outputFilePath);
BufferedOutputStream bufferedOutputStream = new BufferedOutputStream(fileOutputStream);
// 创建Zip输出流
ZipOutputStream zipOutputStream = new ZipOutputStream(bufferedOutputStream);
// 添加Zip实体
ZipEntry zipEntry = new ZipEntry(inputFile.getName());
zipOutputStream.putNextEntry(zipEntry);
// 写入数据
zipOutputStream.write(buffer, 0, count);
// 关闭Zip输出流和文件输出流
zipOutputStream.closeEntry();
zipOutputStream.close();
bufferedOutputStream.close();
fileOutputStream.close();
// 如果当前压缩卷大小大于等于最大卷大小,则增加卷号
if (new File(outputFilePath).length() >= maxVolumeSize) {
volumeIndex++;
}
}
}
}
}
```
该示例代码中,输入文件路径和输出目录路径需要根据实际情况进行替换。在示例代码中,最大卷大小为60MB,可以根据需要进行修改。该示例代码使用了Java中的ZipOutputStream类进行压缩,并在压缩时根据卷大小进行分卷处理。
阅读全文
相关推荐
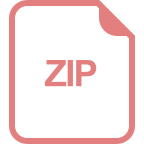
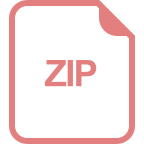
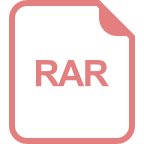
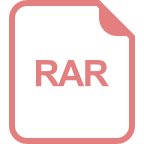
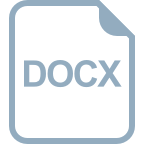
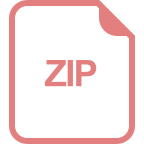
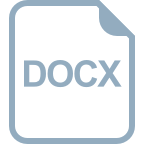
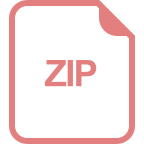