Qt 进行文件组帧,并通过TCP形式从串口中发出文件
时间: 2024-03-02 12:49:20 浏览: 103
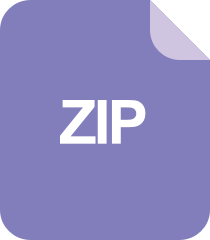
QT通过TCP协议传输文件
使用 Qt 的 QFile 类和 QSerialPort 类可以实现将文件通过串口发送的功能。下面是一个简单的示例代码:
```c++
#include <QFile>
#include <QSerialPort>
#include <QSerialPortInfo>
#include <QCoreApplication>
void sendFileViaSerialPort(QString filePath, QString portName) {
QFile file(filePath);
if (!file.open(QIODevice::ReadOnly)) {
qDebug() << "Failed to open file" << filePath;
return;
}
// 读取文件内容并转换为二进制数据
QByteArray fileData = file.readAll();
// 打开串口
QSerialPort serialPort;
serialPort.setPortName(portName);
serialPort.setBaudRate(QSerialPort::Baud115200);
serialPort.setDataBits(QSerialPort::Data8);
serialPort.setParity(QSerialPort::NoParity);
serialPort.setStopBits(QSerialPort::OneStop);
if (!serialPort.open(QIODevice::ReadWrite)) {
qDebug() << "Failed to open serial port" << portName;
return;
}
// 发送文件内容
qint64 bytesWritten = serialPort.write(fileData);
if (bytesWritten == -1) {
qDebug() << "Failed to write data to serial port";
} else {
qDebug() << "Sent" << bytesWritten << "bytes to serial port";
}
// 关闭串口和文件
serialPort.close();
file.close();
}
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QString filePath = "path/to/file";
QString portName = "COM1";
sendFileViaSerialPort(filePath, portName);
return a.exec();
}
```
上述代码通过 QFile 类读取指定路径的文件内容,然后使用 QSerialPort 类将文件内容通过串口发送。在函数中,首先打开串口并设置串口参数,然后使用 QSerialPort 类的 write() 函数将文件内容写入串口。最后关闭串口和文件。注意,需要在 main 函数中调用 sendFileViaSerialPort() 函数,并提供文件路径和串口名称作为参数。
希望这个示例代码对你有所帮助!
阅读全文
相关推荐



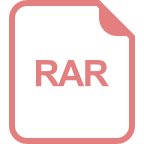
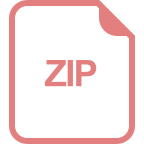
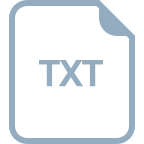
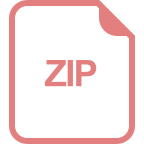
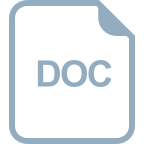





