写一个方法实现静态检查
时间: 2024-01-29 17:04:00 浏览: 22
静态检查是指在代码编译或者静态分析过程中,对代码的语法、规范、风格等方面进行检查。在Java中,可以使用工具如Checkstyle、Findbugs、PMD等来实现静态检查。
下面是一个简单的实现静态检查的例子:
```java
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class StaticCheck {
private final static String REGEX = "\\s(System\\.out\\.println|print(ln)?|(e|E)xit)\\s*\\(";
public static void main(String[] args) {
if (args.length != 1) {
System.out.println("Usage: java StaticCheck <filename>");
System.exit(1);
}
String fileName = args[0];
File file = new File(fileName);
if (!file.exists()) {
System.out.println("File " + fileName + " does not exist");
System.exit(1);
}
try {
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
int lineNumber = 1;
while ((line = reader.readLine()) != null) {
Pattern pattern = Pattern.compile(REGEX);
Matcher matcher = pattern.matcher(line);
if (matcher.find()) {
System.out.println("Found System.out.println, print, println, exit or Exit at line " + lineNumber);
}
lineNumber++;
}
reader.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
这个例子中,我们定义了一个正则表达式来匹配代码中是否存在System.out.println、print、println、exit或Exit语句。然后我们读取传入的文件,逐行进行正则匹配,如果匹配成功就输出对应的提示信息。这样就可以实现一个简单的静态检查了。
当然,在实际的项目中,我们可以使用更为强大的静态检查工具来进行代码检查,例如Checkstyle、Findbugs、PMD等。这些工具可以对代码的规范、质量、性能等方面进行全面的检查,帮助我们提高代码的质量和可维护性。
相关推荐
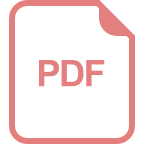
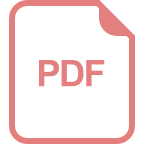
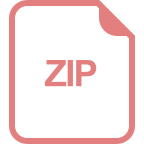














