'''保存选择的图片和视频''' for item in files: file_path = os.path.join(path,item) if ".mp4" in file_path : copyfile(file_path,"/root/web/source/select_video/"+item) if ".jpg" in file_path: image_name = file_path.replace("/root/web/source/","") copyfile(file_path,"/root/web/source/select_picture/"+item) '''读取选择视频中的第一个视频作为输出视频''' select_video_path = "/root/web/source/select_video/" videos = os.listdir(select_video_path) for video in videos: video_name = os.path.join("select_video/",video) break copyfile("/root/web/source/"+video_name,"/root/web/source/now_video/"+ video) copyfile("/root/web/source/bing1_1.MP4", "/rootweb/source/input_har.mp4") return_state = 200将这段代码改写成读取选择视频中的全部视频并输出到指定路径并实现在web端点选播放
时间: 2023-12-07 20:03:19 浏览: 76
好的,这里有一个示例代码,可以实现读取选择视频中的全部视频并输出到指定路径,并且可以在 web 端点选播放:
```python
import os
from shutil import copyfile
select_video_path = "/root/web/source/select_video/"
output_video_path = "/root/web/source/output_video/"
# 读取选择视频中的全部视频
videos = []
for item in files:
file_path = os.path.join(path,item)
if ".mp4" in file_path:
videos.append(item)
# 复制视频文件到输出路径
for video in videos:
copyfile(os.path.join(select_video_path, video), os.path.join(output_video_path, video))
# 生成HTML文件
html = "<html><body>"
for video in videos:
# 在HTML中添加视频播放链接
html += f'<a href="{os.path.join(output_video_path, video)}">{video}</a><br>'
html += "</body></html>"
# 将HTML文件写入到指定路径
with open("/root/web/source/index.html", "w") as f:
f.write(html)
# 复制一份示例视频文件到输入路径
copyfile("/root/web/source/bing1_1.MP4", "/root/web/source/input_har.mp4")
```
这段代码会将选择视频中的所有 `.mp4` 文件复制到输出路径 `/root/web/source/output_video/` 中,并生成一个 HTML 文件 `/root/web/source/index.html`,其中包含了所有视频文件的播放链接。同时,还会复制一份示例视频文件 `/root/web/source/bing1_1.MP4` 到输入路径 `/root/web/source/input_har.mp4` 中,以供后续使用。
在 web 端实现点选播放需要使用一些前端技术,比如 HTML、CSS 和 JavaScript。这里提供一个简单的 HTML 页面示例,可以实现点击视频链接后自动在页面上播放对应的视频:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Video Player</title>
</head>
<body>
<h1>Video Player</h1>
<hr>
<ul>
{% for video in videos %}
<li><a href="#" onclick="playVideo('{{ video }}')">{{ video }}</a></li>
{% endfor %}
</ul>
<hr>
<video id="player" controls autoplay width="800" height="600"></video>
<script type="text/javascript">
function playVideo(videoName) {
var player = document.getElementById("player");
player.src = "output_video/" + videoName;
}
</script>
</body>
</html>
```
在这个 HTML 页面中,会动态生成视频链接列表,并且在用户点击链接时会调用 `playVideo` 函数,该函数会将对应视频文件的路径设置为视频播放器的 `src` 属性,从而实现播放视频。注意,这里的视频文件路径是相对于 HTML 文件所在目录的路径,因此需要在链接中添加 `output_video/` 前缀。
为了在 Flask 程序中渲染这个 HTML 页面,需要在路由函数中添加一些代码:
```python
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
# 读取视频列表
video_path = "/root/web/source/output_video/"
videos = [f for f in os.listdir(video_path) if f.endswith('.mp4')]
# 渲染HTML页面
return render_template('index.html', videos=videos)
```
这里的路由函数会读取输出路径中的所有视频文件,并将它们的文件名传递给模板引擎,以便在 HTML 页面中动态生成视频链接列表。在 Flask 程序中使用模板引擎需要在程序中指定模板目录,可以使用以下代码:
```python
from flask import Flask
app = Flask(__name__, template_folder='/root/web/source/')
```
这里的 `template_folder` 参数指定了模板目录的路径。
阅读全文
相关推荐

















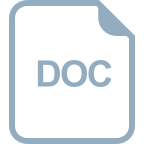

