#!/usr/bin/env python2.7 # coding=UTF-8 import os import sys import cv2 from pyzbar import pyzbar def main(image_folder_path, output_file_name): img_files = [f for f in os.listdir(image_folder_path) if f.endswith(('.png'))] qr_codes_found = [] print("Image files:") for img_file in img_files: print(img_file) for img_file in img_files: img_path = os.path.join(image_folder_path,img_file) img = cv2.imread(img_path) barcodes = pyzbar.decode(img) for barcode in barcodes: if barcode.type == 'QRCODE': qr_data = barcode.data.decode("utf-8") qr_codes_found.append((img_file, qr_data)) with open(output_file_name,'w') as f: for file_name,qr_content in qr_codes_found: file_name = os.path.splitext(file_name)[0] # 去掉文件名的扩展名 f.write("{}: {}\n".format(file_name, qr_content)) if __name__ == "__main__": image_folder_path = '/root/Pictures' output_file_name = 'qr_codes_found.txt' main(image_folder_path,output_file_name)如果识别到相同代码,如何让最后获得的文本中没有相同的解码内容
时间: 2024-04-14 21:25:30 浏览: 14
如果要确保最后获得的文本中没有相同的解码内容,您可以在保存解码结果之前进行一些处理。以下是一种方法:
1. 在`qr_codes_found`列表中保存所有解码结果。
2. 创建一个新的列表`unique_qr_codes`来保存唯一的解码内容。
3. 遍历`qr_codes_found`列表,将每个解码内容添加到`unique_qr_codes`列表中,但仅当该解码内容不在`unique_qr_codes`列表中时才添加。
4. 将`unique_qr_codes`列表中的内容保存到文件中。
下面是修改后的代码示例:
```python
def main(image_folder_path, output_file_name):
img_files = [f for f in os.listdir(image_folder_path) if f.endswith(('.png'))]
qr_codes_found = []
print("Image files:")
for img_file in img_files:
print(img_file)
for img_file in img_files:
img_path = os.path.join(image_folder_path,img_file)
img = cv2.imread(img_path)
barcodes = pyzbar.decode(img)
for barcode in barcodes:
if barcode.type == 'QRCODE':
qr_data = barcode.data.decode("utf-8")
qr_codes_found.append((img_file, qr_data))
unique_qr_codes = []
for file_name, qr_content in qr_codes_found:
if qr_content not in unique_qr_codes:
unique_qr_codes.append(qr_content)
with open(output_file_name,'w') as f:
for qr_content in unique_qr_codes:
f.write("{}\n".format(qr_content))
if __name__ == "__main__":
image_folder_path = '/root/Pictures'
output_file_name = 'qr_codes_found.txt'
main(image_folder_path,output_file_name)
```
这样,最后生成的文本文件中将只包含唯一的解码内容,不会有重复的内容出现。
相关推荐
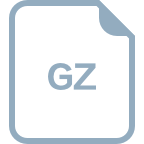
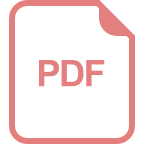
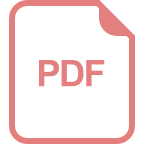















