c++ 使用orb提取两幅图片的特征点并使其分布均匀后,根据特征点信息让这两幅图像进行匹配
时间: 2024-02-03 11:11:48 浏览: 19
使用ORB提取特征点可以使用OpenCV库。以下是一个示例代码,假设有两个输入图像image1和image2:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main(int argc, char** argv)
{
// read images
Mat image1 = imread("path/to/image1.jpg", IMREAD_GRAYSCALE);
Mat image2 = imread("path/to/image2.jpg", IMREAD_GRAYSCALE);
// initialize ORB detector and descriptor
Ptr<ORB> orb = ORB::create();
// detect keypoints
vector<KeyPoint> keypoints1, keypoints2;
orb->detect(image1, keypoints1);
orb->detect(image2, keypoints2);
// compute descriptors
Mat descriptors1, descriptors2;
orb->compute(image1, keypoints1, descriptors1);
orb->compute(image2, keypoints2, descriptors2);
// match descriptors
BFMatcher matcher(NORM_HAMMING);
vector<DMatch> matches;
matcher.match(descriptors1, descriptors2, matches);
// filter matches based on distance
double max_dist = 0;
double min_dist = 100;
for (int i = 0; i < descriptors1.rows; i++)
{
double dist = matches[i].distance;
if (dist < min_dist) min_dist = dist;
if (dist > max_dist) max_dist = dist;
}
vector<DMatch> good_matches;
for (int i = 0; i < descriptors1.rows; i++)
{
if (matches[i].distance <= max(2 * min_dist, 0.02))
{
good_matches.push_back(matches[i]);
}
}
// draw matches
Mat img_matches;
drawMatches(image1, keypoints1, image2, keypoints2,
good_matches, img_matches, Scalar::all(-1), Scalar::all(-1));
// show matches
namedWindow("Matches", WINDOW_NORMAL);
imshow("Matches", img_matches);
waitKey(0);
return 0;
}
```
在这个示例中,ORB检测器和描述符是使用`ORB::create()`初始化的。然后,使用`detect()`方法检测每个图像中的关键点,并使用`compute()`方法计算每个关键点的描述符。接下来,使用`BFMatcher`进行描述符匹配,并使用阈值过滤匹配。最后,使用`drawMatches()`方法绘制匹配,并将其显示在窗口中。
需要注意的是,ORB提取到的特征点可能不会均匀分布,因此可以使用其他方法(如密集采样)来提取均匀分布的特征点。
相关推荐
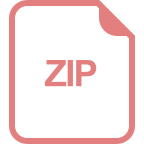
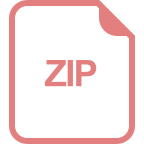
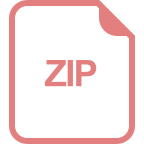













