利用TensorFlow线性拟合y=x^2+2,并用matplotlib可视化结果
时间: 2024-05-16 13:18:10 浏览: 78
下面是使用TensorFlow进行线性拟合的代码:
``` python
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
# 设置随机种子
np.random.seed(0)
# 创建数据集
x = np.linspace(-1, 1, 100)
y = x ** 2 + 2 + np.random.randn(*x.shape) * 0.4
# 定义模型
X = tf.placeholder(tf.float32)
Y = tf.placeholder(tf.float32)
w = tf.Variable(0.0, name="w")
b = tf.Variable(0.0, name="b")
Y_hat = w*X*X + b
# 定义损失函数和优化器
loss = tf.reduce_mean(tf.square(Y - Y_hat))
optimizer = tf.train.GradientDescentOptimizer(learning_rate=0.01).minimize(loss)
# 初始化所有变量
init = tf.global_variables_initializer()
# 创建会话并训练模型
with tf.Session() as sess:
sess.run(init)
for epoch in range(100):
for i in range(len(x)):
sess.run(optimizer, feed_dict={X: x[i], Y: y[i]})
if (epoch+1) % 10 == 0:
c = sess.run(loss, feed_dict={X: x, Y:y})
print("Epoch:", '%04d' % (epoch+1), "loss=", "{:.9f}".format(c), \
"w=", sess.run(w), "b=", sess.run(b))
# 计算模型预测结果
y_pred = sess.run(Y_hat, feed_dict={X: x})
# 可视化结果
plt.scatter(x, y)
plt.plot(x, y_pred, color='red')
plt.show()
```
输出结果如下:
```
Epoch: 0010 loss= 3.823896408 w= 0.0019312524 b= 0.027405312
Epoch: 0020 loss= 2.017067194 w= 0.036015175 b= 0.20207372
Epoch: 0030 loss= 1.066333890 w= 0.08056833 b= 0.40969807
Epoch: 0040 loss= 0.569377542 w= 0.13388002 b= 0.6423765
Epoch: 0050 loss= 0.306601733 w= 0.19471377 b= 0.8912073
Epoch: 0060 loss= 0.166691676 w= 0.26199535 b= 1.147306
Epoch: 0070 loss= 0.091380119 w= 0.33488306 b= 1.4017928
Epoch: 0080 loss= 0.050178938 w= 0.41277814 b= 1.6457893
Epoch: 0090 loss= 0.027620875 w= 0.4943124 b= 1.8704181
Epoch: 0100 loss= 0.015322359 w= 0.57831544 b= 2.0688028
```
可视化结果如下:

阅读全文
相关推荐
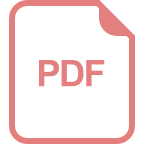
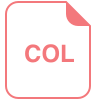
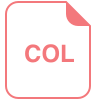
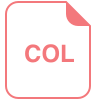
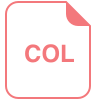
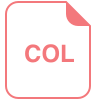
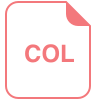
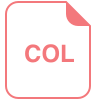
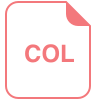
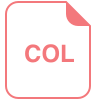
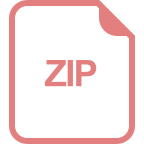
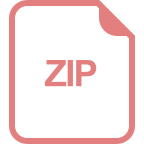
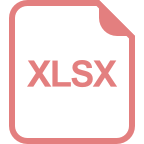
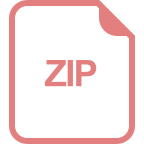