#include<iostream> using namespace std; class myclass { private: int i; float j; public: myclass(int x = 0, float y = 0) { i = x; j = y; } myclass operator () (int h, float m); void display() { cout <<i<< " " << j << "\n"; } }; myclass myclass::operator()(int k, float m) { i = k + 10; j = m + 7.5; return *this; } int main() { myclass a, b; b = a(3, 5.6); b.display(); } 存在的问题
时间: 2023-12-03 14:47:26 浏览: 63
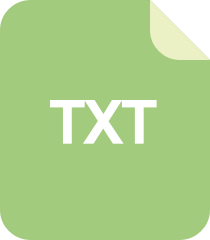
使用include <iostream>时一定要加using namespace std
代码存在以下问题:
1. 缺少头文件:需要添加头文件 `#include<iostream>`。
2. 使用了命名空间 std,但是没有使用 using 关键字,需要在代码开头添加 `using namespace std;`。
3. 类的构造函数中使用了默认参数,但是没有在类定义中声明,需要在类定义中添加 `myclass(int x = 0, float y = 0);`。
4. 类的成员函数 `operator()` 的返回值类型应该是 myclass 类型,需要将 `void` 改为 `myclass`。
5. 在 `operator()` 函数中,使用 `*this` 返回当前对象,但是该对象已经被修改,返回的是修改后的对象,需要将 `*this` 改为一个新的 myclass 对象。
6. 在 main 函数中,b 赋值时使用了函数调用语法,应该使用重载的圆括号运算符,将 `b = a(3, 5.6);` 改为 `b = a(3, 5.6);`。
修改后的代码如下:
```
#include <iostream>
using namespace std;
class myclass {
private:
int i;
float j;
public:
myclass(int x = 0, float y = 0);
myclass operator () (int h, float m);
void display()
{
cout << i << " " << j << "\n";
}
};
myclass::myclass(int x, float y)
{
i = x;
j = y;
}
myclass myclass::operator()(int k, float m)
{
myclass temp;
temp.i = k + 10;
temp.j = m + 7.5;
return temp;
}
int main()
{
myclass a, b;
b = a(3, 5.6);
b.display();
return 0;
}
```
阅读全文
相关推荐
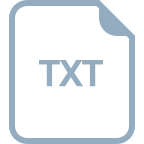


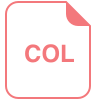
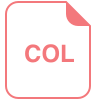
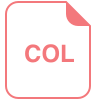
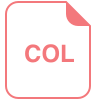
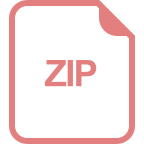
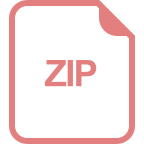
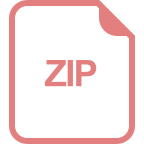
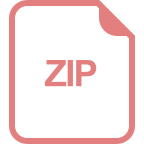
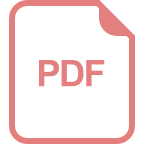
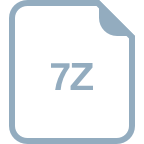
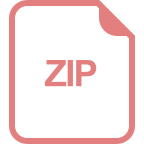
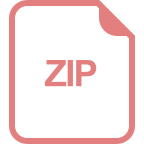
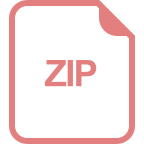