从键盘输入一个字符串到字符数组a中,将其中的大写字符复制到另一个字符数组b中。用C语言
时间: 2023-11-27 09:50:17 浏览: 87
下面是一个简单的C语言程序,可实现输入一个字符串到字符数组a中,将其中的大写字符复制到另一个字符数组b中的功能:
```c
#include <stdio.h>
#include <ctype.h>
#define MAXLEN 100
int main()
{
char a[MAXLEN], b[MAXLEN];
int i, j = 0;
printf("请输入一个字符串:");
fgets(a, MAXLEN, stdin);
for (i = 0; a[i] != '\0'; i++) {
if (isupper(a[i])) {
b[j] = a[i];
j++;
}
}
b[j] = '\0';
printf("大写字符复制到b中的结果为:%s\n", b);
return 0;
}
```
这个程序中,我们使用了C标准库中的`ctype.h`头文件中的`isupper()`函数来判断字符是否为大写字符。`fgets()`函数用于从标准输入(即键盘)读取用户输入的字符串,`MAXLEN`定义了数组a和数组b的最大长度。最后,我们使用了一个循环来遍历数组a中的所有字符,如果该字符是大写字符,则将其复制到数组b中,并将计数器j加1。最后,我们在数组b的末尾加上一个空字符('\0'),以便将其作为字符串输出。
相关问题
通过c语言写从键盘输入一个字符串,将其中的大写字母变成小写字母,其它字符不变。
可以使用C语言内置的字符处理函数toupper()和tolower()实现大写字母转小写字母的功能。
下面是一个示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int i;
printf("Enter a string: ");
fgets(str, 100, stdin); // 从键盘读入字符串
for (i = 0; str[i] != '\0'; i++) {
if (isupper(str[i])) { // 判断是否为大写字母
str[i] = tolower(str[i]); // 转换成小写字母
}
}
printf("Lowercase string: %s", str);
return 0;
}
```
注意,使用fgets()函数可以避免输入字符串时出现缓冲区溢出的问题。
从键盘输入一个字符串,将字符串中的大写字母转换成小写字母后进行输出的功能。C语言
可以使用 C 语言中的库函数 `tolower()` 将大写字母转换成小写字母。下面是一个示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int i;
printf("请输入一个字符串:");
fgets(str, 100, stdin);
for (i = 0; str[i] != '\0'; i++) {
if (isupper(str[i])) {
str[i] = tolower(str[i]);
}
}
printf("转换后的字符串为:%s", str);
return 0;
}
```
在上面的代码中,使用了 `fgets()` 函数从键盘输入一个字符串,然后使用 `for` 循环遍历字符串中的每个字符,如果该字符是大写字母,则使用 `tolower()` 函数将其转换成小写字母。最后输出转换后的字符串。
相关推荐
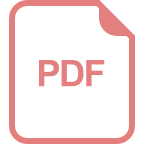
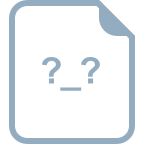
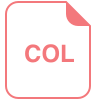
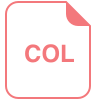
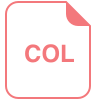
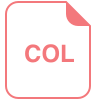
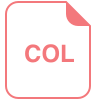







