linux C h264文件转MP4文件 代码
时间: 2023-12-22 11:05:47 浏览: 31
以下是一个简单的示例程序,使用FFmpeg库将H.264视频文件转换为MP4文件:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <libavformat/avformat.h>
#include <libavcodec/avcodec.h>
#include <libavutil/avutil.h>
#include <libavutil/opt.h>
int main(int argc, char *argv[]) {
AVFormatContext *in_fmt_ctx = NULL, *out_fmt_ctx = NULL;
AVCodecContext *codec_ctx = NULL;
AVCodec *codec = NULL;
AVPacket pkt;
AVFrame *frame = NULL;
int ret, i;
if (argc < 3) {
printf("Usage: %s <input_file.h264> <output_file.mp4>\n", argv[0]);
return -1;
}
// Register all the codecs and formats
av_register_all();
// Open input file
if ((ret = avformat_open_input(&in_fmt_ctx, argv[1], NULL, NULL)) < 0) {
printf("Error: Could not open input file '%s'\n", argv[1]);
return ret;
}
// Retrieve input stream information
if ((ret = avformat_find_stream_info(in_fmt_ctx, NULL)) < 0) {
printf("Error: Could not find stream information\n");
return ret;
}
// Open output file
if ((ret = avformat_alloc_output_context2(&out_fmt_ctx, NULL, NULL, argv[2])) < 0) {
printf("Error: Could not create output context\n");
return ret;
}
// Find codec
codec = avcodec_find_encoder(AV_CODEC_ID_H264);
if (!codec) {
printf("Error: Could not find H.264 codec\n");
return -1;
}
// Add video stream to output context
AVStream *out_stream = avformat_new_stream(out_fmt_ctx, codec);
if (!out_stream) {
printf("Error: Could not create video stream\n");
return -1;
}
out_stream->codecpar->codec_id = codec->id;
// Copy codec parameters from input stream to output stream
AVStream *in_stream = in_fmt_ctx->streams[0];
codec_ctx = avcodec_alloc_context3(codec);
avcodec_parameters_to_context(codec_ctx, in_stream->codecpar);
codec_ctx->codec_tag = 0;
if (out_fmt_ctx->oformat->flags & AVFMT_GLOBALHEADER)
codec_ctx->flags |= AV_CODEC_FLAG_GLOBAL_HEADER;
// Open codec
if ((ret = avcodec_open2(codec_ctx, codec, NULL)) < 0) {
printf("Error: Could not open codec\n");
return ret;
}
// Allocate frame
frame = av_frame_alloc();
if (!frame) {
printf("Error: Could not allocate frame\n");
return -1;
}
// Initialize packet
av_init_packet(&pkt);
pkt.data = NULL;
pkt.size = 0;
// Write header to output file
avformat_write_header(out_fmt_ctx, NULL);
// Read frames from input file and write to output file
while (av_read_frame(in_fmt_ctx, &pkt) >= 0) {
if (pkt.stream_index == 0) {
// Decode packet
ret = avcodec_send_packet(codec_ctx, &pkt);
if (ret < 0) {
printf("Error: Could not send packet for decoding\n");
return ret;
}
while (ret >= 0) {
ret = avcodec_receive_frame(codec_ctx, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
break;
else if (ret < 0) {
printf("Error: Could not decode frame\n");
return ret;
}
// Encode frame
AVPacket enc_pkt;
av_init_packet(&enc_pkt);
enc_pkt.data = NULL;
enc_pkt.size = 0;
ret = avcodec_send_frame(codec_ctx, frame);
if (ret < 0) {
printf("Error: Could not send frame for encoding\n");
return ret;
}
while (ret >= 0) {
ret = avcodec_receive_packet(codec_ctx, &enc_pkt);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
break;
else if (ret < 0) {
printf("Error: Could not encode packet\n");
return ret;
}
// Write packet to output file
av_write_frame(out_fmt_ctx, &enc_pkt);
av_packet_unref(&enc_pkt);
}
}
}
av_packet_unref(&pkt);
}
// Write trailer to output file
av_write_trailer(out_fmt_ctx);
// Free resources
avcodec_free_context(&codec_ctx);
av_frame_free(&frame);
avformat_close_input(&in_fmt_ctx);
avio_closep(&out_fmt_ctx->pb);
avformat_free_context(out_fmt_ctx);
return 0;
}
```
编译命令如下:
```
gcc -o h264_to_mp4 h264_to_mp4.c -lavformat -lavcodec -lavutil
```
使用示例:
```
./h264_to_mp4 input.h264 output.mp4
```
相关推荐
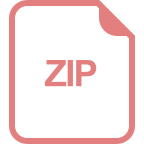
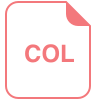
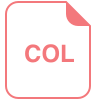
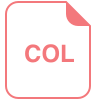
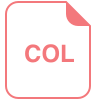
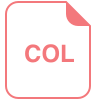









