请制作一段c++代码,作用是生成一个俄罗斯方块游戏程序
时间: 2023-03-13 10:29:08 浏览: 129
我不太清楚你想要的程序到底是什么样子,但是我可以尝试为你编写一段C代码:
#include <stdio.h>
int main()
{
int i, j;
for (i = 0; i < 10; i++)
{
for (j = 0; j < 10; j++)
{
if (i == 0 || i == 9 || j == 0 || j == 9)
printf("*");
else
printf(" ");
}
printf("\n");
}
return 0;
}
相关问题
c++俄罗斯方块游戏代码
根据引用中的代码,可以看出这段代码是用来初始化游戏数据的。使用了memset函数将player、preBlock和tetrisMap这几个变量的内存内容全部初始化为0。同时,给player结构体中的ttime成员赋值为当前系统时间。这段代码的主要作用是准备游戏数据,为后续的俄罗斯方块游戏提供基础。
引用中给出了游戏中使用到的玩家数据结构GAME_TETRIS的定义。其中包含了一些游戏中需要记录的信息,如计时、分数、当前方块类型等。这个结构体用来保存玩家的游戏状态。
引用中的代码片段是俄罗斯方块游戏中用来将预生成的随机方块载入游戏地图的代码。在代码中,首先判断player结构体中的newBlockFlag标识是否为真,如果是真则执行后续操作。接着,将预生成的方块载入游戏地图,然后进行消除满行方块、生成新方块和判断游戏是否结束等操作。这段代码实现了俄罗斯方块游戏中方块的移动和消除等功能。
综上所述,这些引用代码片段是俄罗斯方块游戏的一部分,包括了游戏数据的初始化和方块的载入、移动和消除等操作。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [c/c++ 实现俄罗斯方块小游戏(附全部源码)](https://blog.csdn.net/q841447739/article/details/126249698)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
写一个俄罗斯方块c++代码
好的,以下是一个简单的俄罗斯方块的C++代码,仅供参考:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <conio.h>
using namespace std;
const int WIDTH = 10; // 画布宽度
const int HEIGHT = 20; // 画布高度
// 俄罗斯方块类
class Tetromino {
public:
Tetromino(int type, int x = 0, int y = 0)
: type(type), x(x), y(y) {
// 初始化方块矩阵
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
matrix[i][j] = false;
}
}
switch (type) {
case 0: // I 形
matrix[1][0] = true;
matrix[1][1] = true;
matrix[1][2] = true;
matrix[1][3] = true;
break;
case 1: // J 形
matrix[0][0] = true;
matrix[1][0] = true;
matrix[1][1] = true;
matrix[1][2] = true;
break;
case 2: // L 形
matrix[0][2] = true;
matrix[1][0] = true;
matrix[1][1] = true;
matrix[1][2] = true;
break;
case 3: // O 形
matrix[0][0] = true;
matrix[0][1] = true;
matrix[1][0] = true;
matrix[1][1] = true;
break;
case 4: // S 形
matrix[0][1] = true;
matrix[0][2] = true;
matrix[1][0] = true;
matrix[1][1] = true;
break;
case 5: // T 形
matrix[0][1] = true;
matrix[1][0] = true;
matrix[1][1] = true;
matrix[1][2] = true;
break;
case 6: // Z 形
matrix[0][0] = true;
matrix[0][1] = true;
matrix[1][1] = true;
matrix[1][2] = true;
break;
}
}
// 获取方块矩阵
bool getMatrix(int row, int col) const {
return matrix[row][col];
}
// 获取方块类型
int getType() const {
return type;
}
// 获取方块位置
int getX() const {
return x;
}
int getY() const {
return y;
}
// 将方块向左移动
void moveLeft() {
x--;
}
// 将方块向右移动
void moveRight() {
x++;
}
// 将方块向下移动
void moveDown() {
y++;
}
// 将方块逆时针旋转
void rotate() {
bool temp[4][4];
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
temp[i][j] = matrix[i][j];
}
}
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
matrix[i][j] = temp[j][3 - i];
}
}
}
private:
bool matrix[4][4]; // 方块矩阵
int type; // 方块类型
int x, y; // 方块位置
};
// 画布类
class Canvas {
public:
Canvas() {
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (j == 0 || j == WIDTH - 1 || i == HEIGHT - 1) {
data[i][j] = true;
} else {
data[i][j] = false;
}
}
}
}
// 判断方块是否可以放置
bool canPlace(const Tetromino& tetromino) const {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (tetromino.getMatrix(i, j)) {
int row = tetromino.getY() + i;
int col = tetromino.getX() + j;
if (data[row][col]) {
return false;
}
}
}
}
return true;
}
// 放置方块
void place(const Tetromino& tetromino) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (tetromino.getMatrix(i, j)) {
int row = tetromino.getY() + i;
int col = tetromino.getX() + j;
data[row][col] = true;
}
}
}
}
// 消除已满行
int clearFullRows() {
int count = 0;
for (int i = HEIGHT - 2; i > 0; i--) {
bool full = true;
for (int j = 1; j < WIDTH - 1; j++) {
if (!data[i][j]) {
full = false;
break;
}
}
if (full) {
count++;
for (int k = i; k > 0; k--) {
for (int j = 1; j < WIDTH - 1; j++) {
data[k][j] = data[k - 1][j];
}
}
i++;
}
}
return count;
}
// 输出画布
void print() const {
system("cls");
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (data[i][j]) {
cout << "#";
} else {
cout << " ";
}
}
cout << endl;
}
}
private:
bool data[HEIGHT][WIDTH]; // 画布数据
};
int main() {
srand(time(NULL)); // 初始化随机数种子
Canvas canvas; // 创建画布对象
Tetromino tetromino(rand() % 7); // 随机生成一个俄罗斯方块
int score = 0; // 分数
while (true) {
canvas.print(); // 输出画布
cout << "Score: " << score << endl;
if (!canvas.canPlace(tetromino)) { // 如果方块不能放置
canvas.place(tetromino); // 将方块放置到画布上
int count = canvas.clearFullRows(); // 消除已满行
score += count * 10; // 根据消除的行数计算分数
tetromino = Tetromino(rand() % 7); // 随机生成一个新的俄罗斯方块
if (!canvas.canPlace(tetromino)) { // 如果新的方块也不能放置
break; // 游戏结束
}
}
if (_kbhit()) { // 如果有按键输入
char ch = _getch();
if (ch == 'a') { // 将方块向左移动
Tetromino temp = tetromino;
temp.moveLeft();
if (canvas.canPlace(temp)) {
tetromino = temp;
}
} else if (ch == 'd') { // 将方块向右移动
Tetromino temp = tetromino;
temp.moveRight();
if (canvas.canPlace(temp)) {
tetromino = temp;
}
} else if (ch == 's') { // 将方块向下移动
Tetromino temp = tetromino;
temp.moveDown();
if (canvas.canPlace(temp)) {
tetromino = temp;
}
} else if (ch == 'w') { // 将方块逆时针旋转
Tetromino temp = tetromino;
temp.rotate();
if (canvas.canPlace(temp)) {
tetromino = temp;
}
}
}
tetromino.moveDown(); // 将方块向下移动
Sleep(100); // 等待一段时间
}
cout << "Game over! Your score is " << score << endl;
return 0;
}
```
这只是一个简单的俄罗斯方块游戏,如果你想要更加完整的游戏体验,可以尝试添加音效、计时、排行榜等功能。
阅读全文
相关推荐





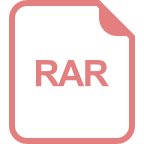
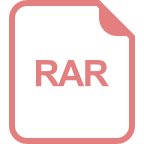
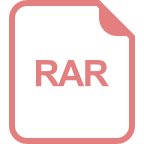








